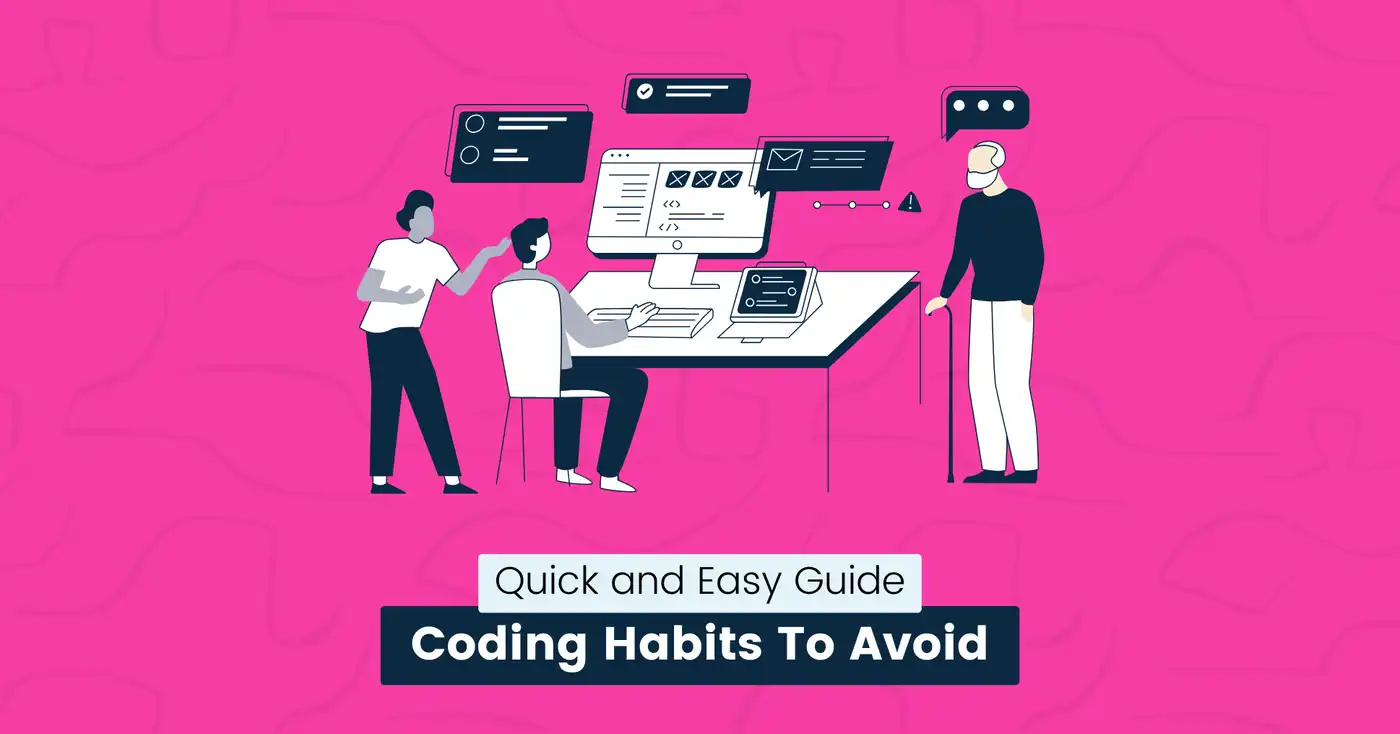
11 Coding Habits To Avoid in 2025
Did you know that most coding errors arise from simple, avoidable habits?
Many programmers understand the importance of good practices, but some still repeat mistakes that lead to software crashes and security breaches that cost clients money and damage their reputations.
In fact, inefficient coding practices can slow down software performance by up to 70%.
These bad coding habits clash with modern tech demands, prompting developers to ignore dependency checks, implement weak logic, or prioritize speed above accessibility.
While such shortcuts may work initially, they eventually result in systems that leak data, have unverifiable API endpoints, and fail to execute on mobile devices.
So, how do you avoid these problems?
The fix starts with recognizing that how you code matters more than ever.
This guide breaks down the 11 bad habits that are putting your code at risk and explains how to eliminate them before competitors do.
You will learn practical tips to stop repeating these mistakes and improve your coding style.
Let’s get started.
Copy-Paste Without Abstraction
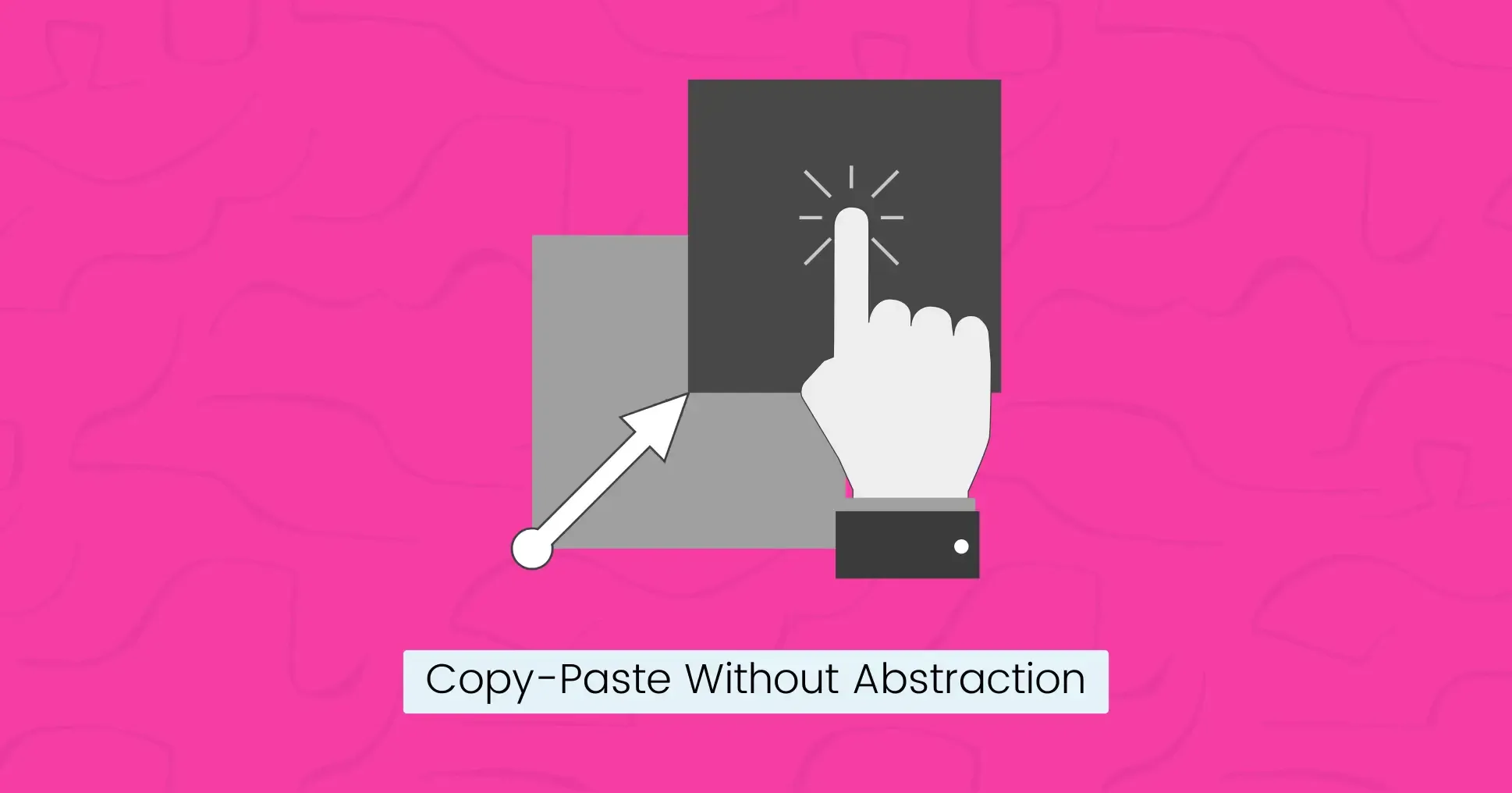
When developers copy and paste code instead of abstracting similar logic into reusable functions or components, they repeat the same code in multiple places.
This approach goes against the “Don’t Repeat Yourself” (DRY) principle.
Not to mention that repeatedly pasting the same code, such as form validation, API calls, or UI elements, creates invisible debt.
When requirements change, you’ll need to hunt down every duplicate instance, increasing the risk of errors or inconsistent behavior.
Why It’s Bad:
- A bug fix in one copy won’t pass on to others.
- Small variations across copies (for example, different error messages for similar forms) might make things more complex.
- It also makes the codebase larger and harder to maintain and debug, which slows down development.
How to Fix It:
- Identify Patterns Early: If you’re pasting code for the third time, extract that logic into a separate function or module.
- Use Parameterization: Turn copied blocks into functions that accept arguments to handle slight changes.
- Centralize Shared Logic: Move reused business rules to a shared service or custom hook.
- Conduct Code Reviews: Regular code reviews help catch instances of duplication early.
- Use Automated Tools: Use code analysis tools that highlight duplicate code.
snappify will help you to create
stunning presentations and videos.
Over-Engineering Simple Solutions
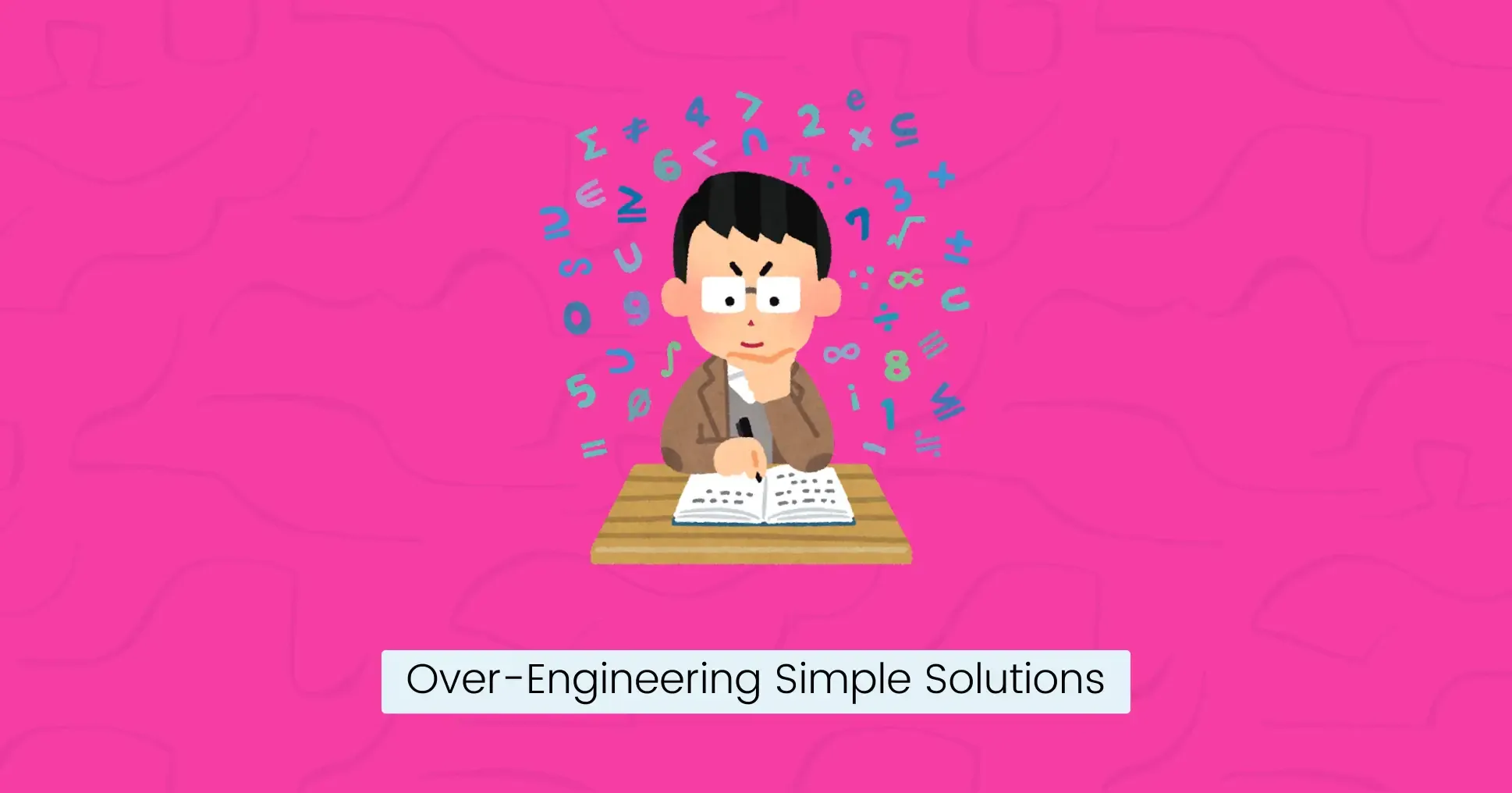
Over-engineering occurs when developers use advanced patterns or complicated logic for simple tasks.
This adds layers of complexity that provide no real value but create future maintenance traps.
Why It’s Bad:
- It can lead to a codebase that is hard to understand and maintain.
- Over-abstracted code becomes rigid. Adding a simple feature might require modifying 5 files.
- It increases cognitive load, and new team members waste time-solving nonexistent problems.
How to Fix It:
- Solve Current Problems: Build the simplest working version first.
- Follow the YAGNI Principle: Only build necessary features, not based on hypothetical future requirements.
- Validate Before Abstracting: Don’t create a generic component until you have 3+ scenarios with shared logic.
Poor Naming Conventions
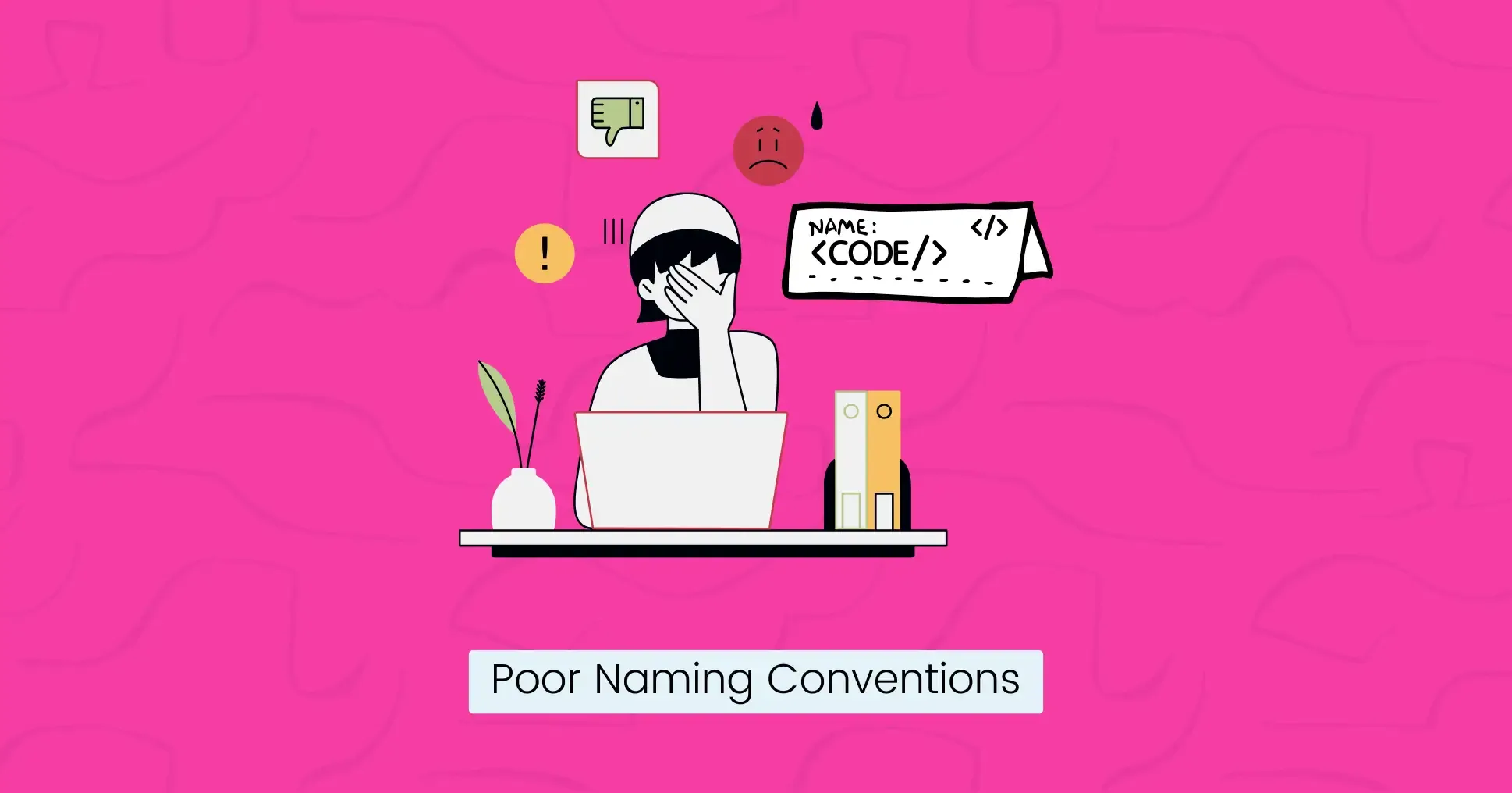
A name should make its purpose obvious without requiring context.
Using vague names forces developers to guess what a variable, function, or class represents, slows debugging, and makes collaboration painful.
If you’re tempted to write a comment to explain a variable, rewrite the variable instead.
Why It’s Bad:
- A poorly named function might hide critical side effects, leading to unintended bugs when reused.
- Generic names make it hard to locate specific logic in large codebases.
How to Fix It:
- Be Descriptive: Choose names that clearly describe the variable’s or function’s purpose.
- Follow Conventional Rules: Use camelCase or PascalCase across your codebase.
- Avoid Abbreviations: Use full words or common abbreviations only when your team universally understands them.
Overuse of Global Variables
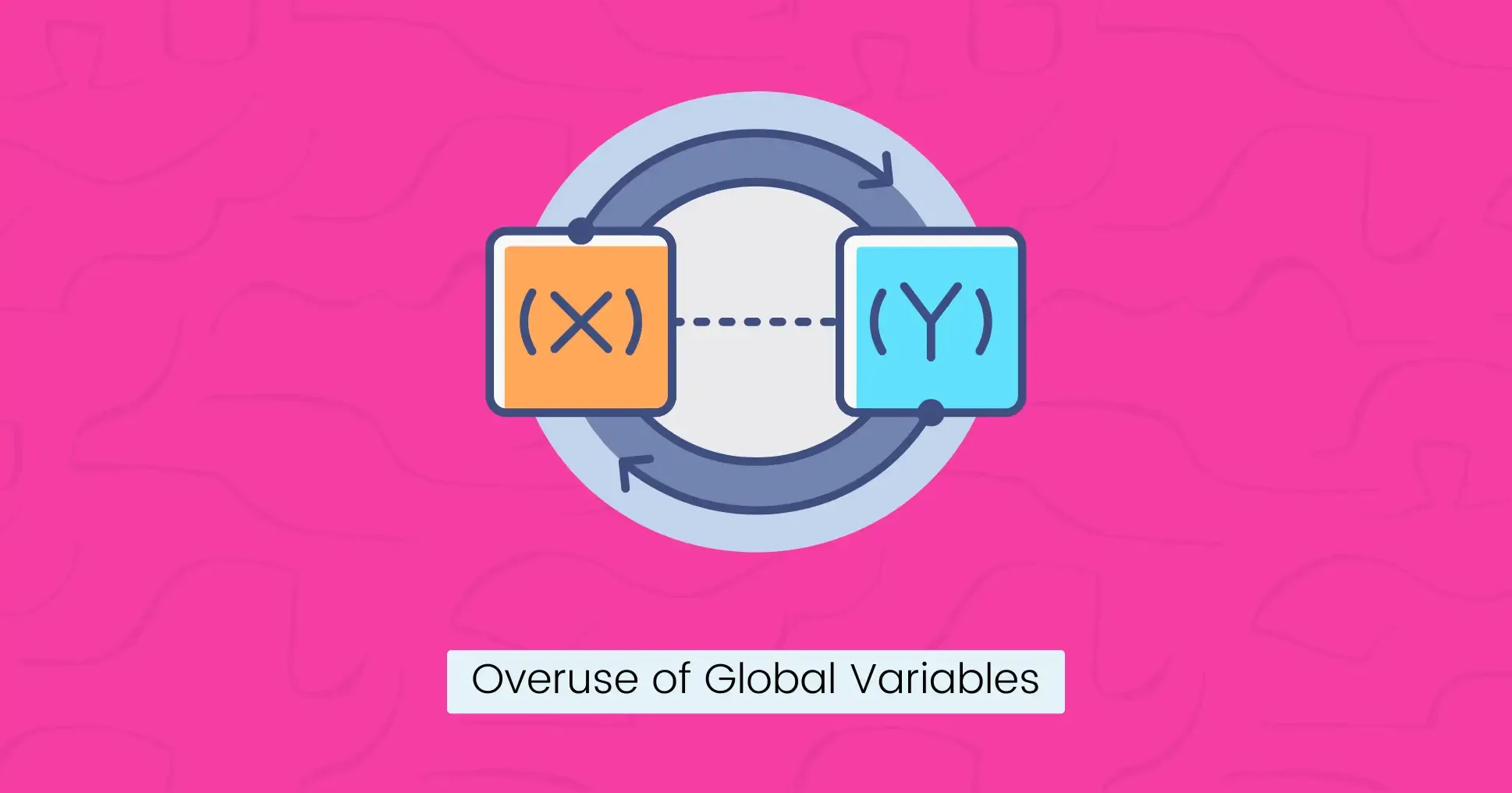
Global variables are declared in a scope that makes them accessible from any part of your application.
While this can seem convenient for shared data, it often leads to developers overusing them, causing unintended modifications and hidden dependencies.
Why It’s Bad:
- Any function or script can alter a global variable, making bugs hard to trace.
- Global scope risks conflicts between your variables and third-party scripts.
- Tests relying on the global state become challenging, as changes in one test can affect others.
How to Fix It:
- Encapsulate State: Group related data and functions into classes or modules to control access.
- Use Closures: Restrict variable access to functions that need it.
- Dependency Injection: Pass shared data explicitly as function arguments.
Writing Monolithic Functions
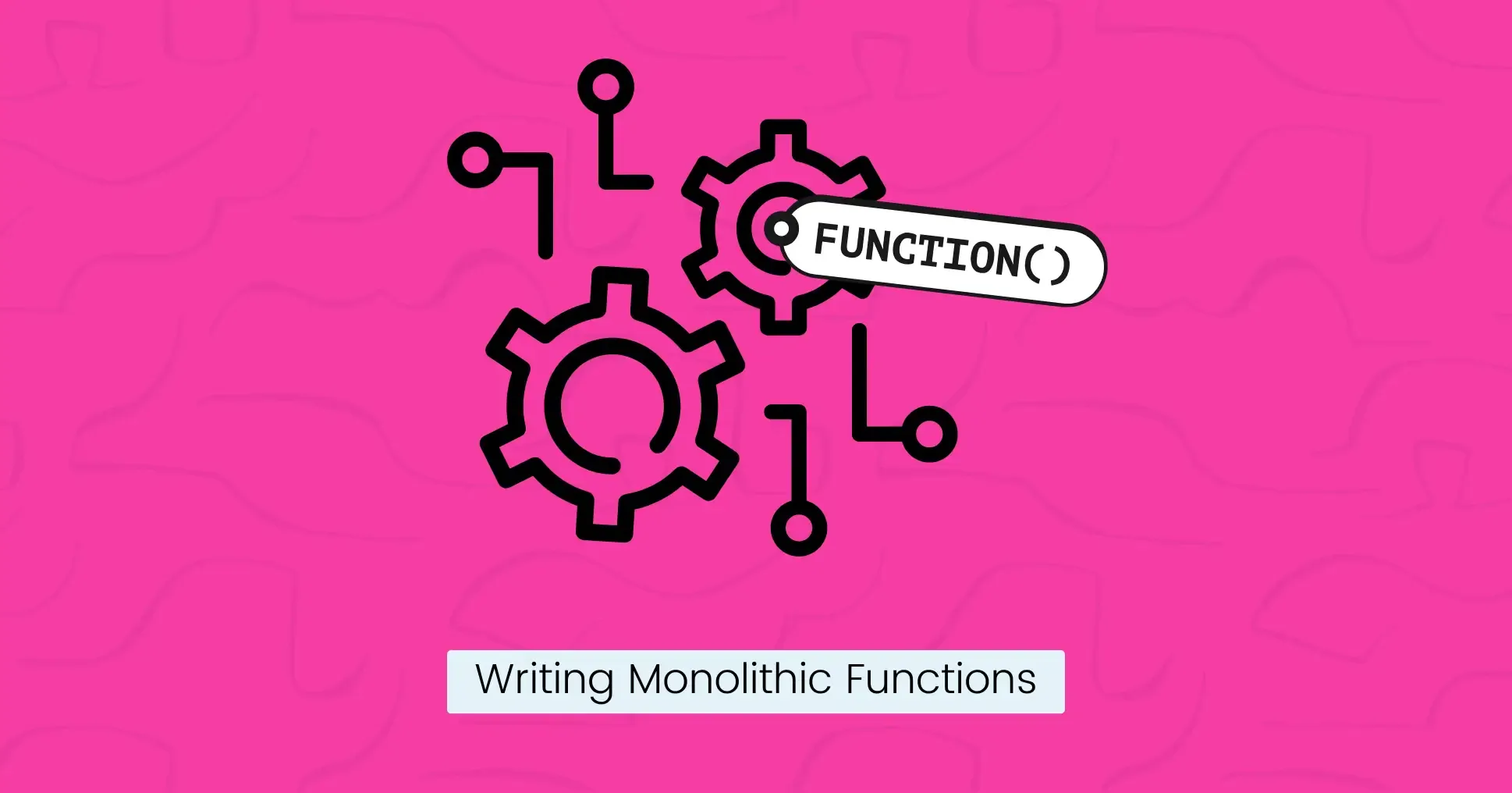
Creating functions that handle multiple unrelated tasks in a single block of code makes them unreadable, hard to test, and resistant to change.
A function should be small enough that you can grasp its purpose in 5 seconds.
If you can’t, it’s already too complex.
Why It’s Bad:
- A change to one part of the function risks breaking unrelated logic.
- Critical logic buried inside a monolithic function can’t be reused elsewhere without copy-pasting.
- Unit tests become difficult, requiring dozens of mocks to cover all side effects.
How to Fix It:
- Single-Responsibility Principle: Split functions into smaller units that do one thing.
- Extract Conditionals: Move complex if/else blocks into separate functions with descriptive names.
Shallow Documentation and Code Comments
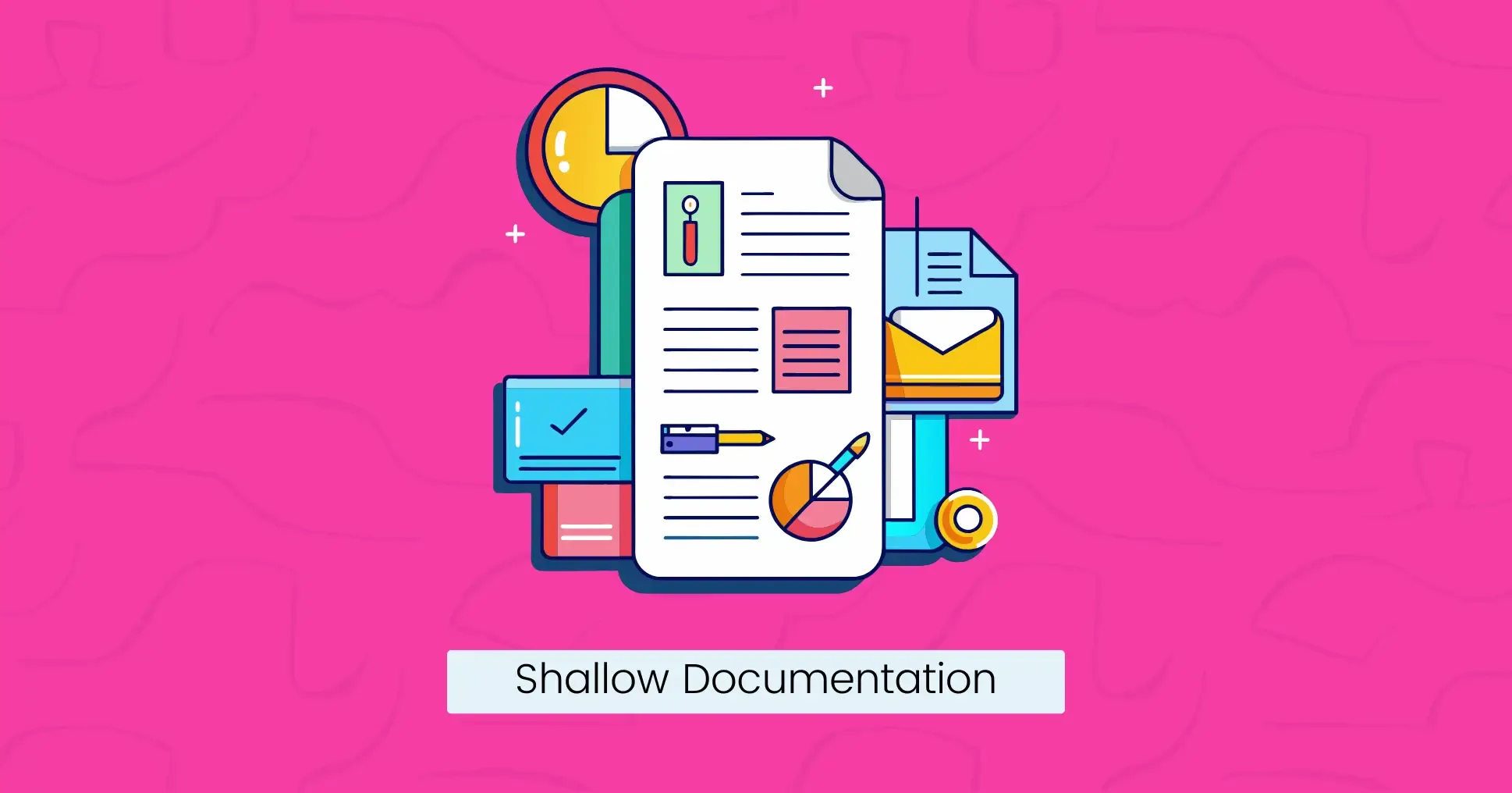
Sometimes, developers write comments that only describe what the code is doing rather than explaining its reason.
They fail to capture critical context like trade-offs, past decisions, or edge cases influencing the implementation.
Why It’s Bad:
- Future maintainers can’t differentiate between intentional design and accidental workarounds.
- The lack of detailed explanations makes it difficult to assess the impact of changes or integrate new features.
How to Fix It:
- Avoid Obvious Statements: Delete comments that paraphrase code and focus on context that cannot be understood from the code itself.
- Document the Why: Replace useless comments with explanations of decision criteria.
- Review Docs: Update docs and comments regularly. Reject PRs with comments that only describe the mechanics.
Inefficient Error Handling
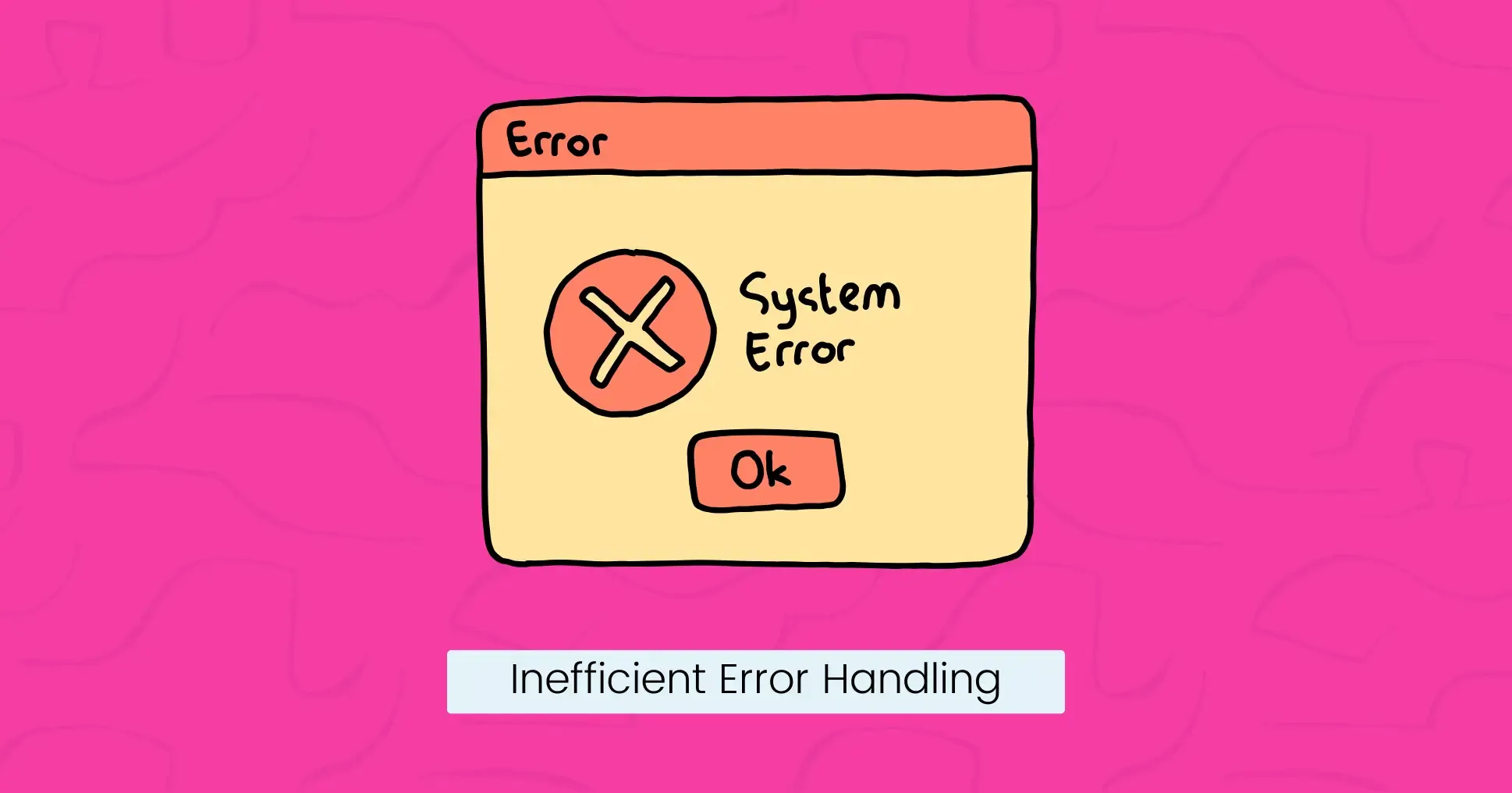
Errors are common in web development. Even good programmers with years of experience make mistakes.
However, not catching errors where they occur or catching them but not doing anything useful leaves systems unstable.
Why It’s Bad:
- Unhandled errors corrupt data and leave processes in invalid states.
- Generic notifications like “something went wrong” lack context, forcing teams to reverse-engineer problems.
How to Fix It:
- Classify Errors: Categorize issues based on severity and priority.
- Context-Based Logging: Attach metadata (user ID, API endpoint, timestamp) to error logs for faster resolution.
Over-Reliance on Frameworks
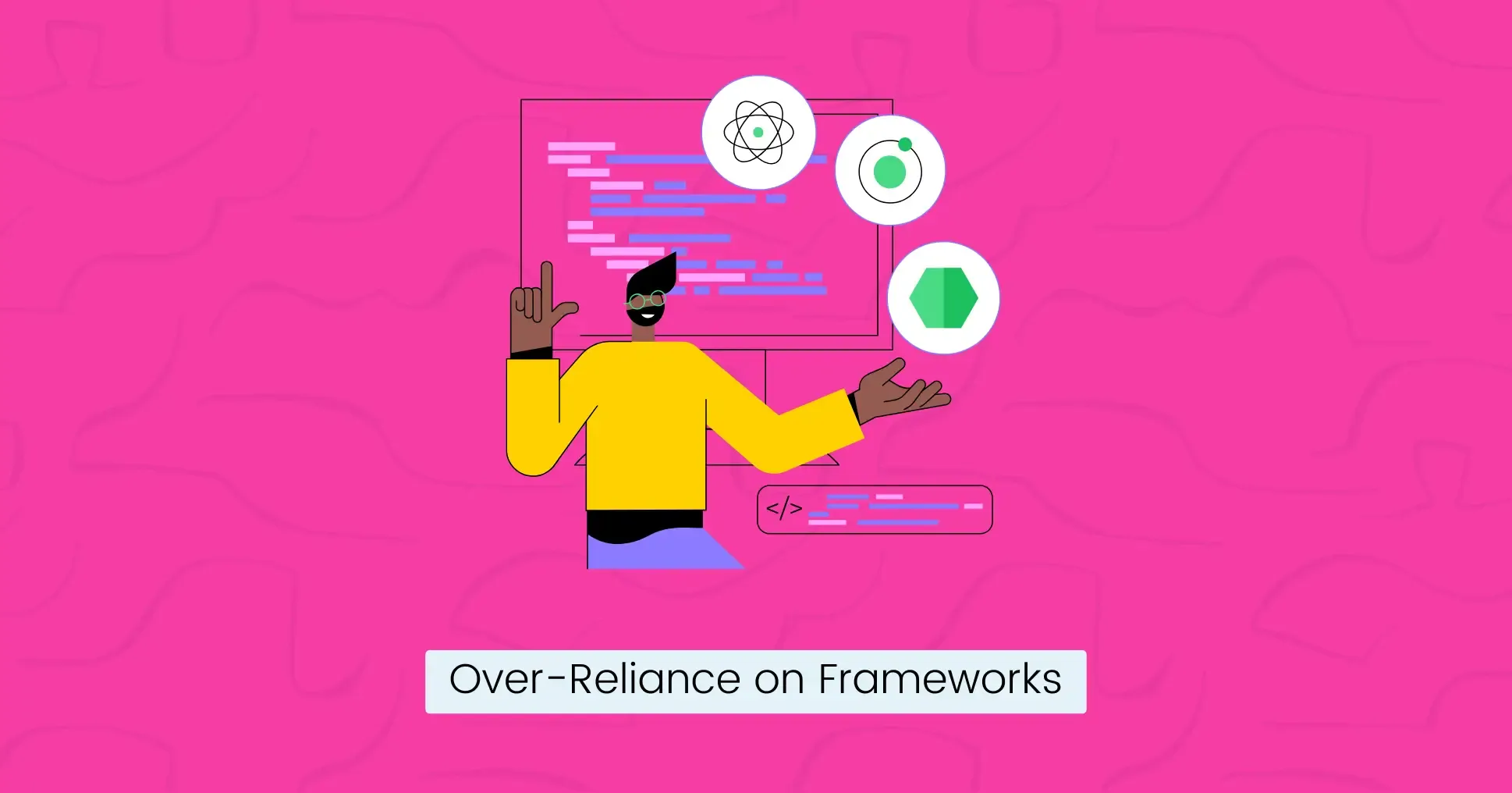
Web development frameworks can speed up development but are not a replacement for core knowledge.
Depending too much on frameworks without learning the underlying principles may cause you to forget the fundamentals.
For example, React developers do not understand the DOM or virtual DOM mechanics.
Why It’s Bad:
- Errors in framework-specific code become impossible to comprehend without knowledge of essential principles such as browser render cycles.
- It makes it harder to adapt to new requirements or replace components in your stack when necessary.
How to Fix It:
- Learn the Basics First: Invest time in understanding core programming concepts behind your chosen frameworks. For example, if using React, study browser APIs and DOM manipulation.
- Read the Framework’s Documentation: Study its core architecture and features, not just usage examples.
- Build Framework-Agnostic Modules: Separate business logic (authentication, data transforms) into pure functions or classes that work independently.
Neglecting Security
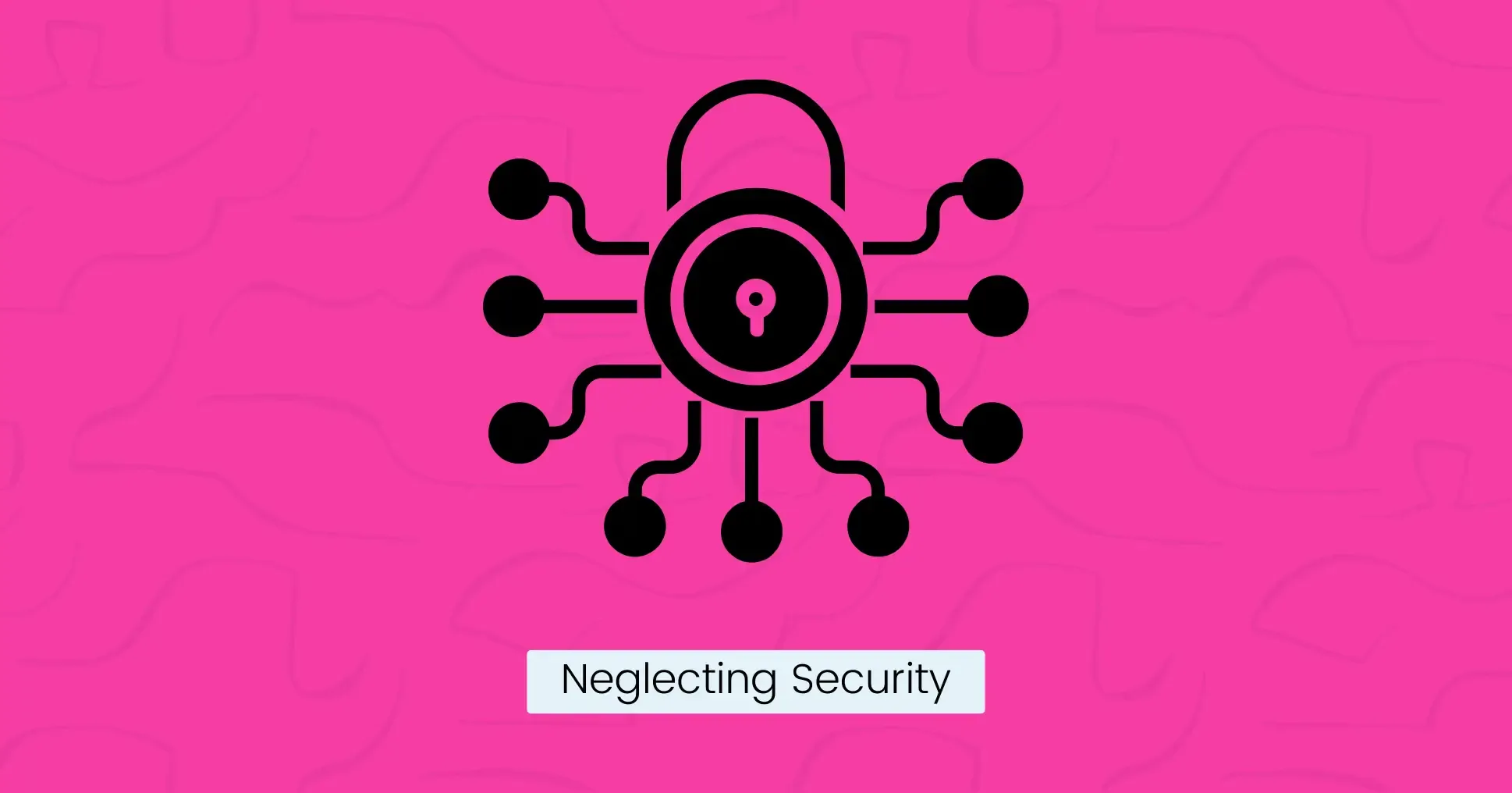
Skipping security best practices during development creates vulnerable gaps that turn minor mistakes into major breaches.
This happens when developers take shortcuts by hardcoding sensitive information, not validating user input, or ignoring dependency vulnerabilities.
Why It’s Bad:
- Hardcoded credentials can be easily extracted, allowing unauthorized access.
- Unfiltered user input (forms, URLs) enables SQLi, XSS, or command execution.
- Outdated dependencies become easy entry points for malware.
- Data breaches result in legal penalties and lost customer trust.
How to Fix It:
- Validate All Inputs: Apply strict input validation schemas for all external data like APIs, forms, and cookies.
- Regular Dependency Updates: Keep libraries and dependencies up to date by monitoring for security patches.
Treating AI-generated Code as Production-Ready
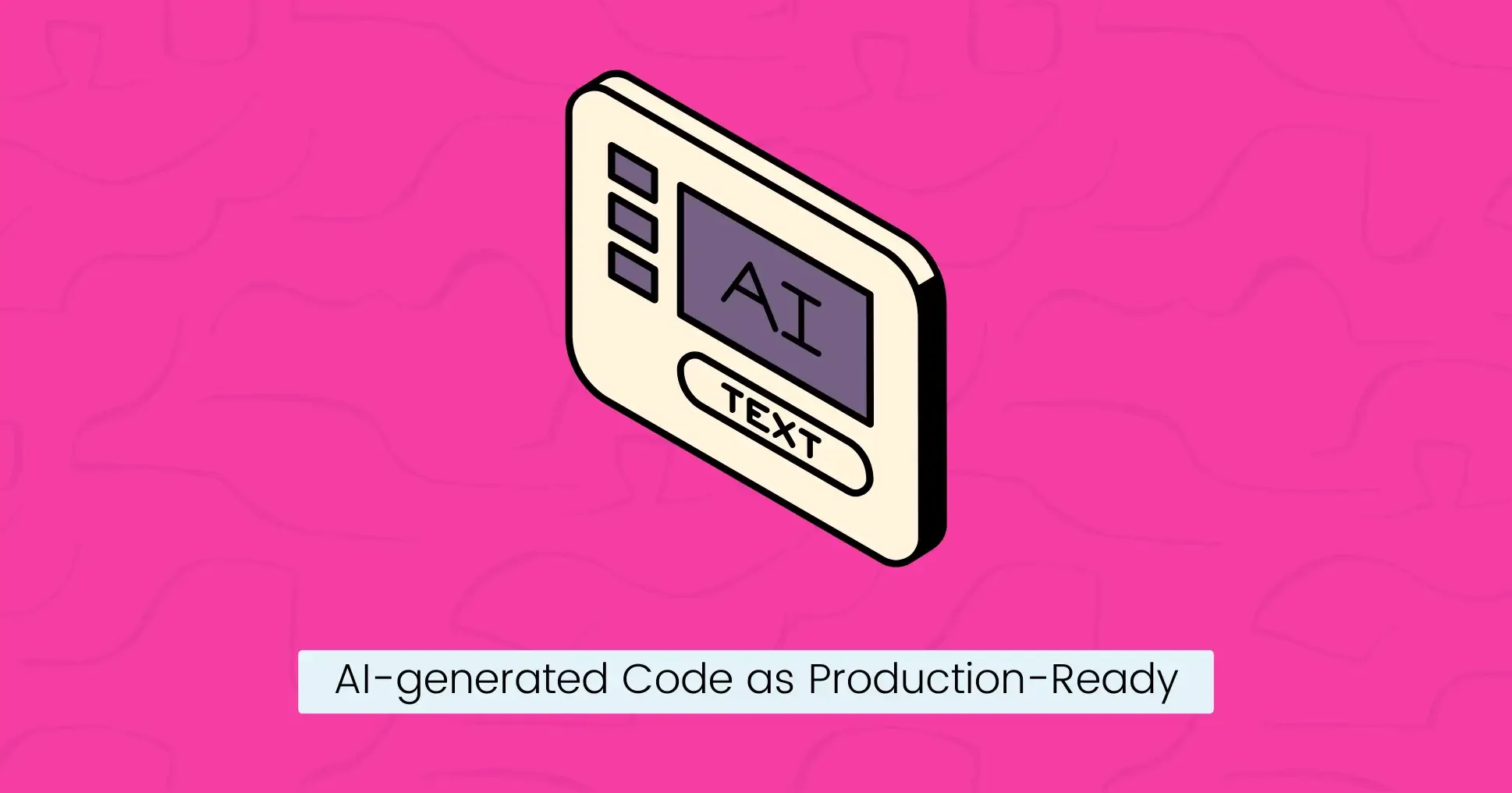
AI tools are changing how developers work by providing intelligent solutions to write code faster, automating repetitive tasks, and increasing productivity.
However, relying too much on AI-generated code from tools like Copilot or ChatGPT without proper review introduces bugs.
AI tools prioritize syntax over context, often ignoring your codebase’s unique error-handling rules, legacy systems, or performance limitations.
Why It’s Bad:
- AI code looks correct but may use outdated APIs or insecure practices.
- AI often adds unnecessary hooks or redundant logic, increasing bundle size.
How to Fix It:
- Treat AI Code as a Draft: Never copy-paste directly. Rewrite suggestions to fit your codebase’s style and standards.
- Manual Validation: Conduct code reviews for AI-generated code.
- Limit AI’s Scope: Use AI only for boilerplate code or documentation, not critical logic.
Poor Time Management and Rushing to Meet Deadlines
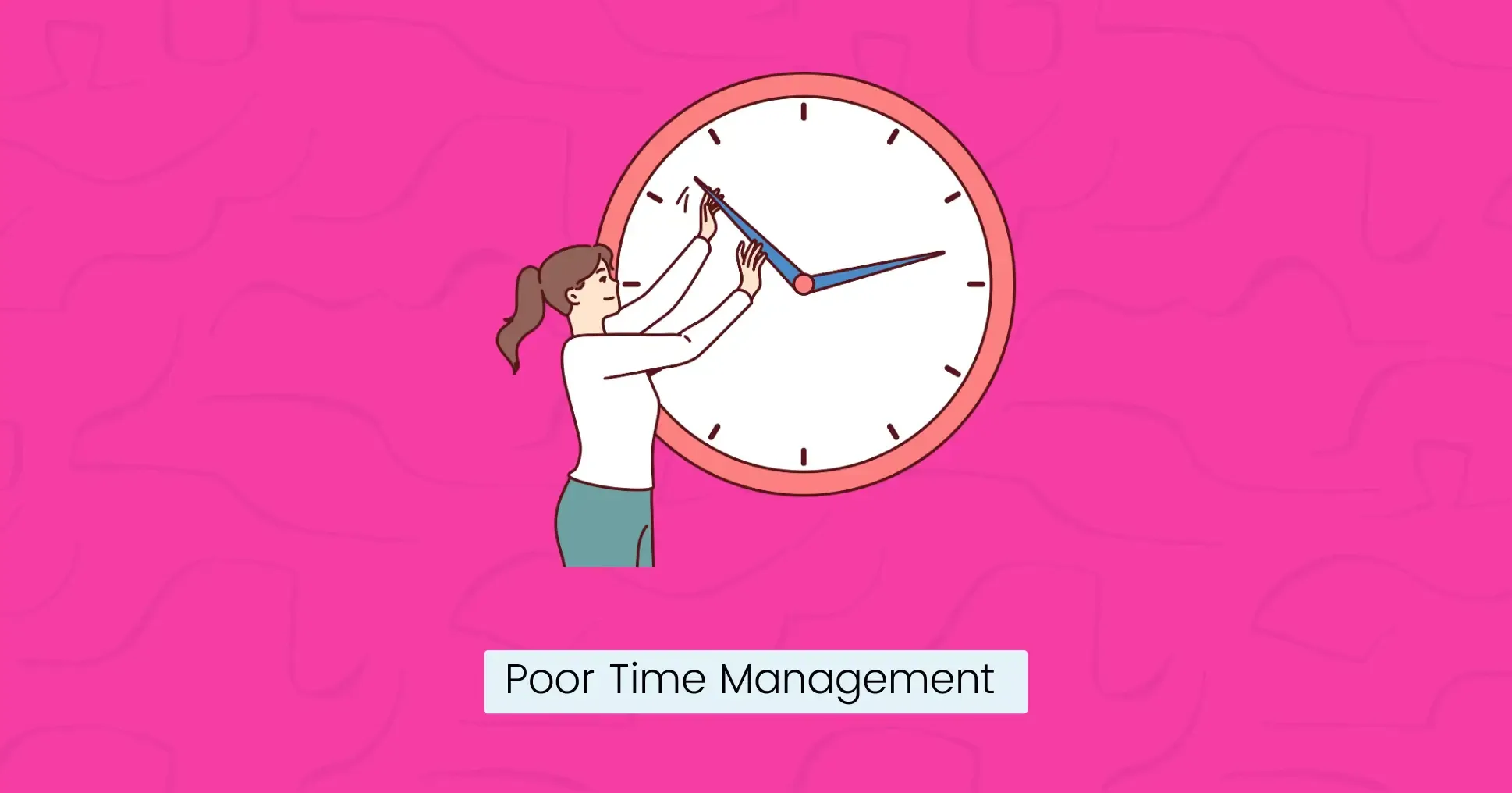
Prioritizing speed over quality to save time leads to technical debt, security oversights, and burnout.
Developers might skip writing tests, bypass code reviews, or ignore best practices to meet a deadline. This can result in unreliable software that fails under real-world use.
Why It’s Bad:
- Quick fixes today become week-long debugging sessions later.
- Rushed work delivers features that meet deadlines but not user needs.
- You become mentally and physically worn out and start making more mistakes.
How to Fix It:
- Set Goals: Break tasks into 2-4 hour blocks with clear goals.
- Save Refactoring Time: Allocate 10-15% of each sprint to address tech debt from rushed work. Automate repetitive tasks to free up development hours.
snappify will help you to create
stunning presentations and videos.
Final Words
As a coder, you must be aware of these harmful habits and actively avoid them for a more productive development experience.
This way, you can build good habits that will help you improve your coding skills and become a 10x developer.
FAQs:
What are the coding habits that improve collaborative development?
Use consistent coding standards, write self-documenting functions, and conduct regular code reviews to ensure clarity and improve teamwork.
What steps can you take to shift away from bad coding habits?
Start by auditing your current code, pinpoint areas for improvement, and set up regular feedback sessions to guide gradual and sustainable improvement.