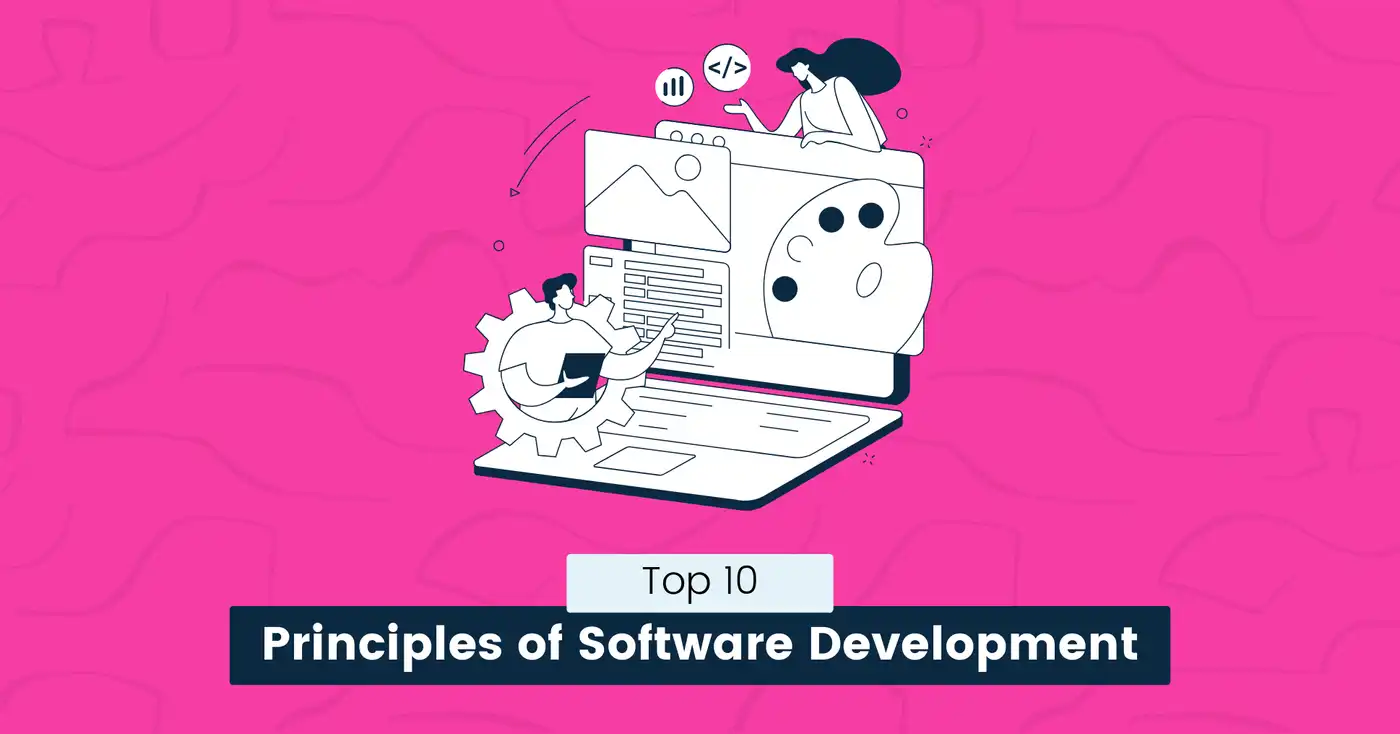
10 Principles of Software Development (2024)
Developers need clear rules and guidelines to build quality software.
Following established principles can make the difference between a software project’s success and failure.
Software development principles help create reliable, fast, secure software that meets user expectations.
They provide a structured approach to software system design and reduce the time and effort required for successful project completion.
This guide will help you learn the core principles of software development and how to implement them to improve your coding efficiency.
Let’s get started.
Why Are Software Development Principles Important?
Principles in software development are essential guidelines that help simplify the process.
They are best practices and concepts that guide developers in making informed decisions about design and implementation.
Here are some advantages of sticking to these principles:
- Consistency: Principles provide a consistent approach to coding, which helps maintain quality and uniformity across the codebase and design system.
- Easy Maintenance: Updating software over time is much easier when a set of rules is followed. Modular code is easier to read and maintain, so there is less chance of new defects being introduced.
- Performance Optimization: Clear guidelines help build software that performs well under increasing loads and can be efficiently managed.
- Risk Mitigation: Following best security practices helps protect the software from vulnerabilities and security threats. They help identify and mitigate risks early in the development process.
- Collaborative Development: They improve team member’s ability to communicate and work together, which leads to improved decision-making and problem-solving.
- Cost-Effective: By catching bugs early and following best practices, developers can avoid costly fixes later.
- Improved User Experience: By following principles, developers can build software that solves real-world problems and meets the needs and expectations of its users.
snappify will help you to create
stunning presentations and videos.
10 Principles of Software Development
Let’s look at the key software development principles you should incorporate into your daily workflows.
KISS (Keep It Simple, Stupid)
.BXBZTnCw_Z2a5NhL.webp)
Software engineering involves complex system designs, features, and interactions between components.
Complex code often introduces more bugs, which makes debugging more stressful. This can lead to confusion and increased development time.
The idea of the KISS principle is to keep things simple, straightforward, and clear.
By keeping things simple, you create easier to maintain and update software over time.
To achieve simplicity in the software development process, developers should:
- Write clean code that is easier to read and use.
- Divide a big problem into smaller, manageable parts.
- Avoid over-engineering and only add features that are necessary to meet system requirements.
- Use clear names for variables, functions, and classes.
DRY (Don’t Repeat Yourself)
.pLMvxZq7_6tSRp.webp)
Software maintenance can become a nightmare if your project contains repetitive code.
Every time you need to update a piece of code, you must track down every instance across your codebase. If you miss one, a new bug is introduced.
This wastes time and increases the risk of introducing new errors.
DRY principle emphasizes the importance of avoiding code duplication and encourages writing reusable code.
Instead of writing the same code in multiple places, write it once and reuse it wherever needed. This way, you can get many benefits like:
- Eliminate redundancy and keep your code clean.
- Make your code consistent and maintainable.
- Create reusable functions, modules, and classes to simplify your development process.
- Make testing easier and faster.
Architecture-First Approach
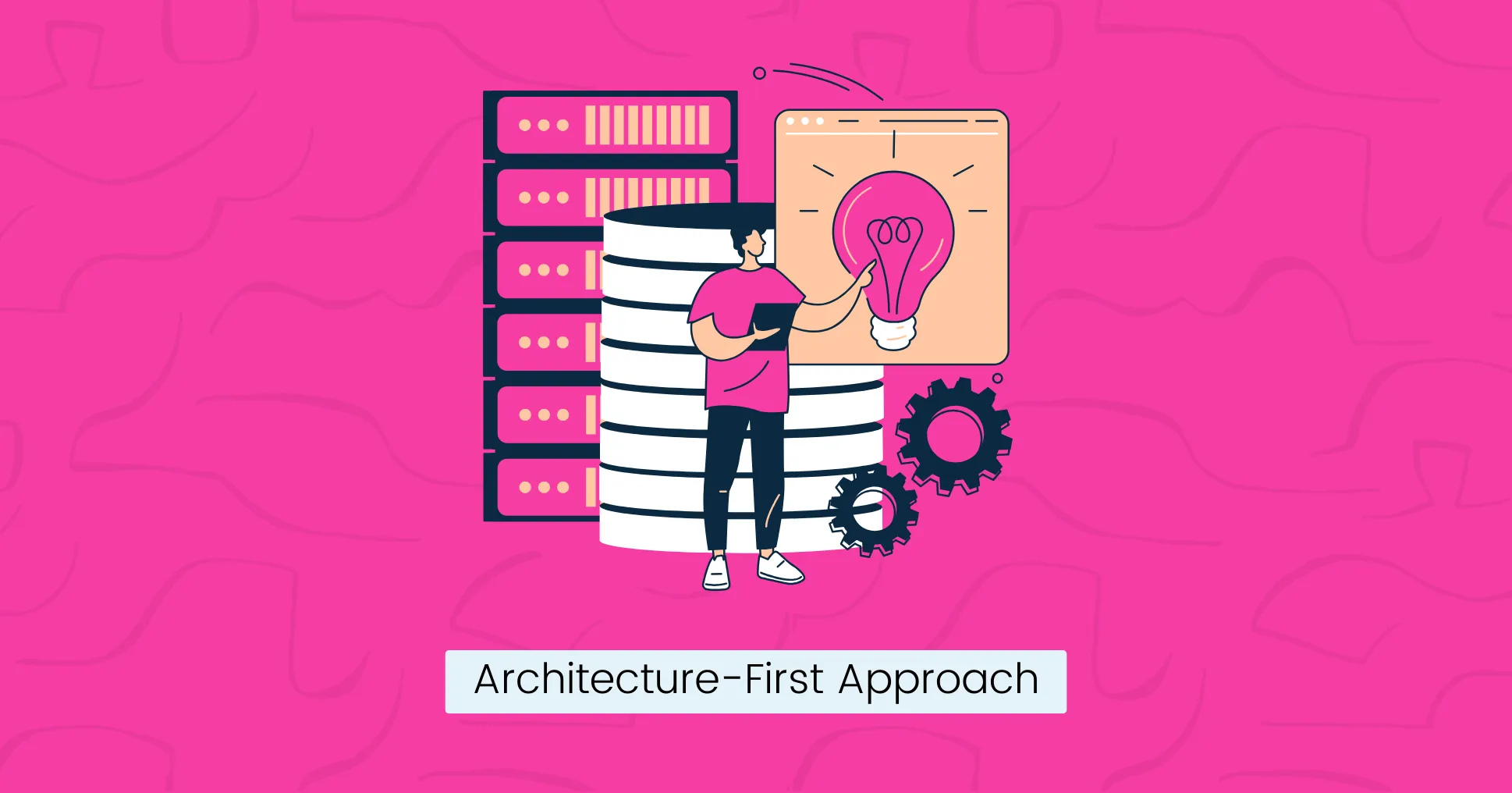
If you start developing a website without a clear plan, it can lead to a disorganized codebase.
As your project grows, adding new features and addressing performance issues becomes harder.
Using the architecture-first approach, you can build a strong foundation that will support your project’s evolving requirements.
This strategy ensures your project has a solid structure, making it easier to develop, manage, and scale.
Moreover, an architectural design improves performance by identifying potential issues early.
YAGNI (You Ain’t Gonna Need It)
.-NsTmdXp_1lE4Rt.webp)
When you build features that are not immediately needed, you waste time and resources that could be better used to improve existing functionality.
These extra features can complicate your code and add new bugs.
YAGNI principle focuses on building only what is required right now, without worrying about what features might be needed in the future.
By prioritizing the current requirements, developers can get benefits such as:
- Deliver a quality product on time.
- Keep code simpler and easier to manage.
- Resource optimization.
Agile Methodology
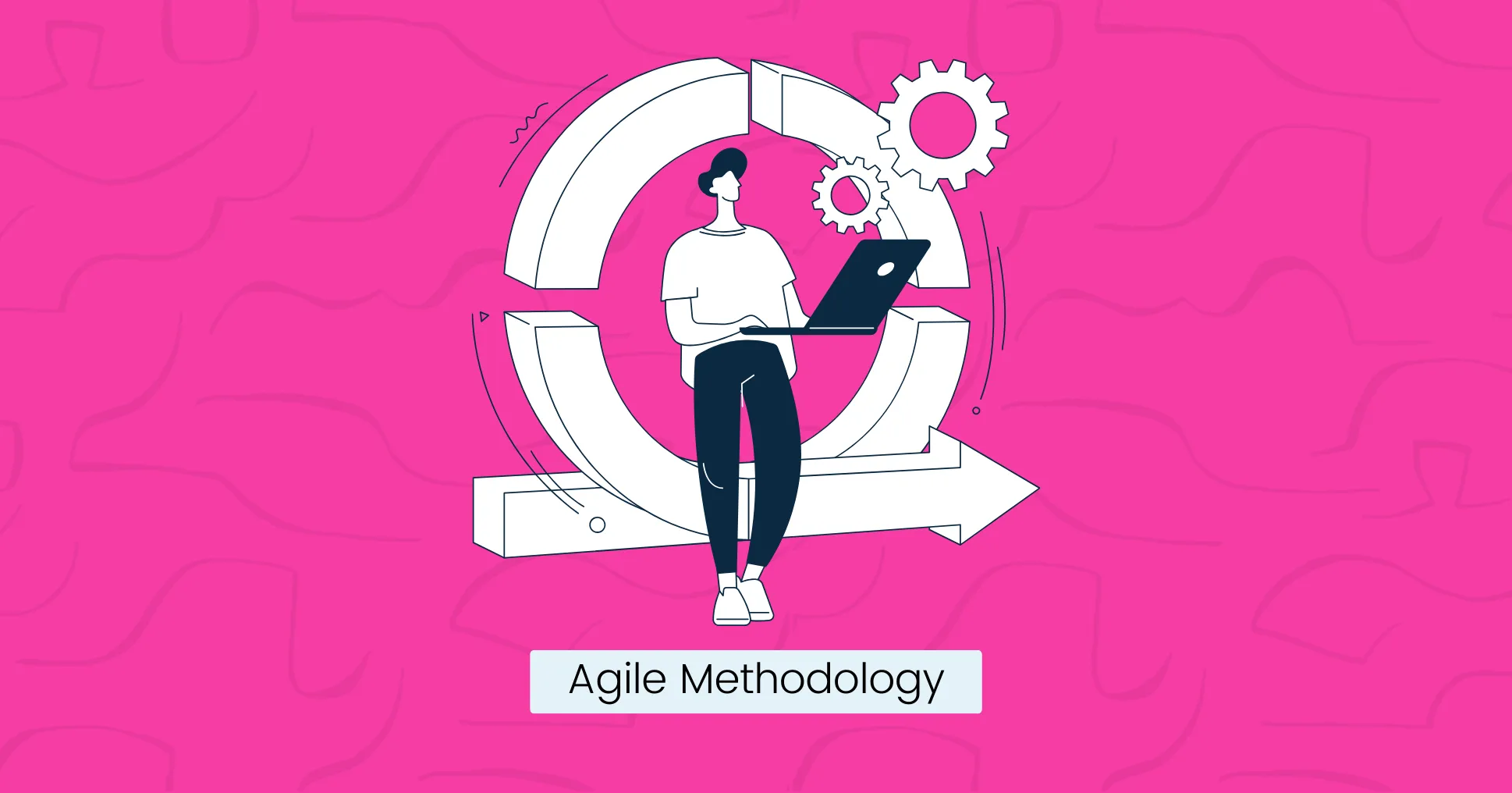
Conventional project management might result in software not being updated to meet user’s needs and missing deadlines.
Agile software development is a flexible and iterative approach that splits complex projects into several manageable tasks.
It focuses on regularly releasing small, functional pieces of the software and using feedback to improve the product continuously.
By adopting Agile methodology, developers can:
- Adapt to changing requirements quickly.
- Easily manage and execute project plans.
- Deliver high-quality software products on time.
- Improve customer satisfaction.
Kanban and Scrum are the two most popular methods used in Agile development.
User-Centered Design
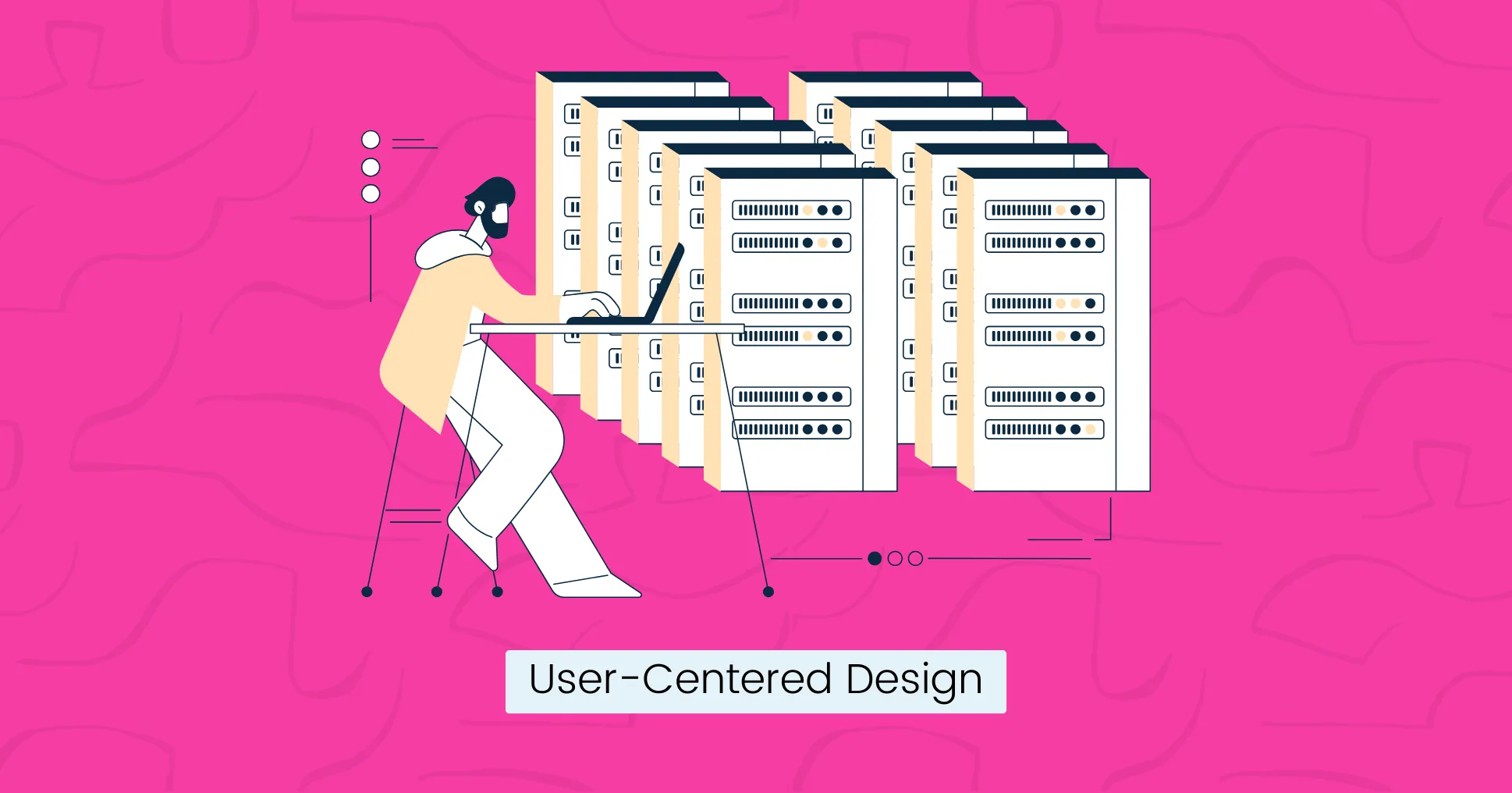
Imagine working day and night on a product users don’t want to use.
Software developed without a clear understanding of users can negatively impact a brand’s reputation.
Users struggle to find and use key features due to complicated interfaces, resulting in low adoption and negative feedback.
User-centered design prioritizes the needs of end users at every stage of the design process.
By focusing on the user experience, you create products that are easy and enjoyable to use.
Iterative Development Lifecycle
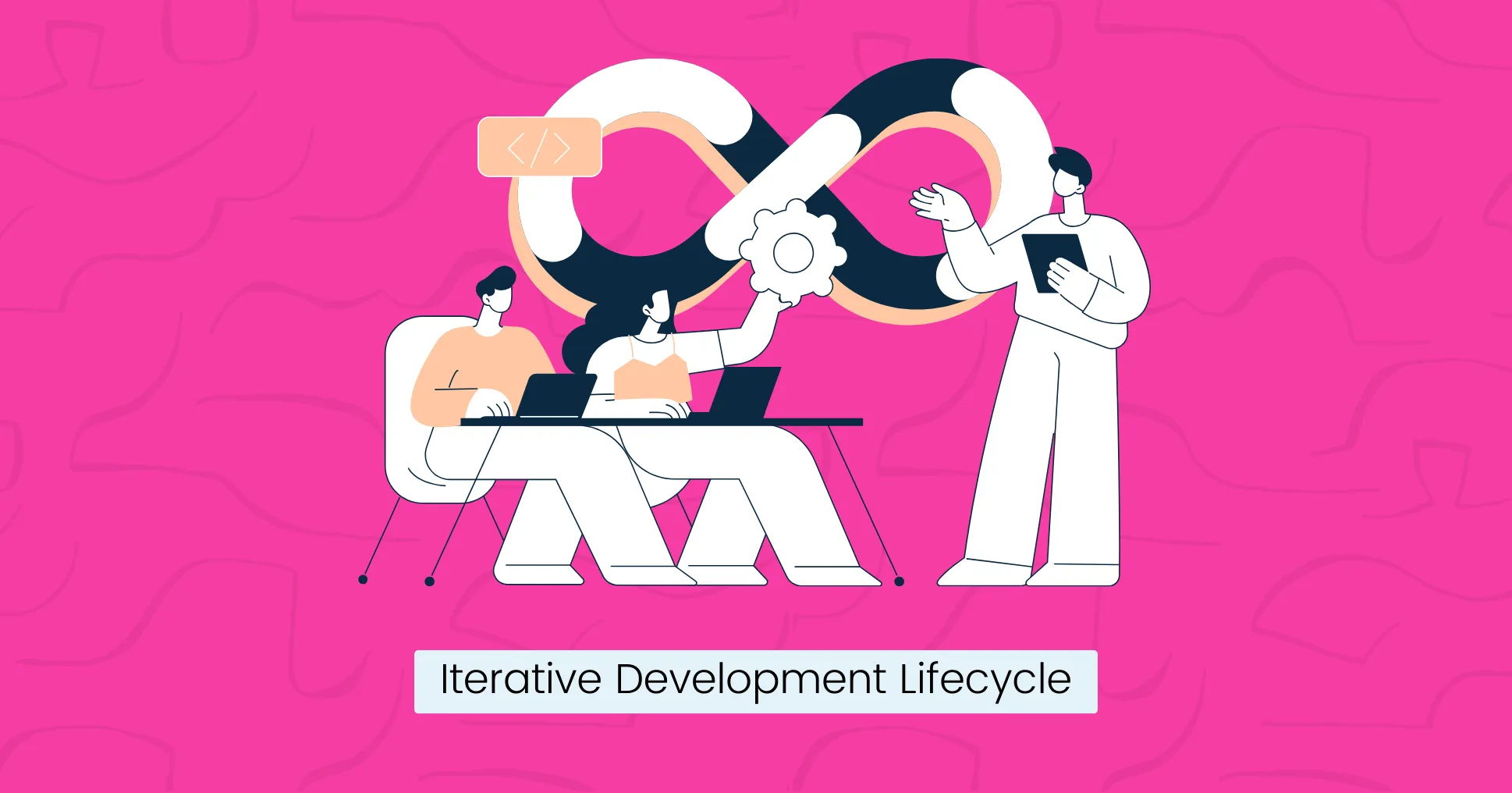
Long development cycles often require extensive modifications, wasting time and resources.
They also delay feedback, so critical issues are not noticed until late.
The iterative life cycle process involves continuously reviewing requirements, design, implementation, and testing to improve the quality of the software.
It involves breaking down the development process into small, manageable iterations or cycles, allowing for frequent evaluation and adaptation to changing requirements.
The iterative life cycle process has many benefits:
- Easily adapt to growing user expectations.
- Identify and fix problems early in the development process.
- Continuous feedback and improvement ensure that the software remains relevant for a long time.
- Faster time to market functional software.
Component-Based Approach
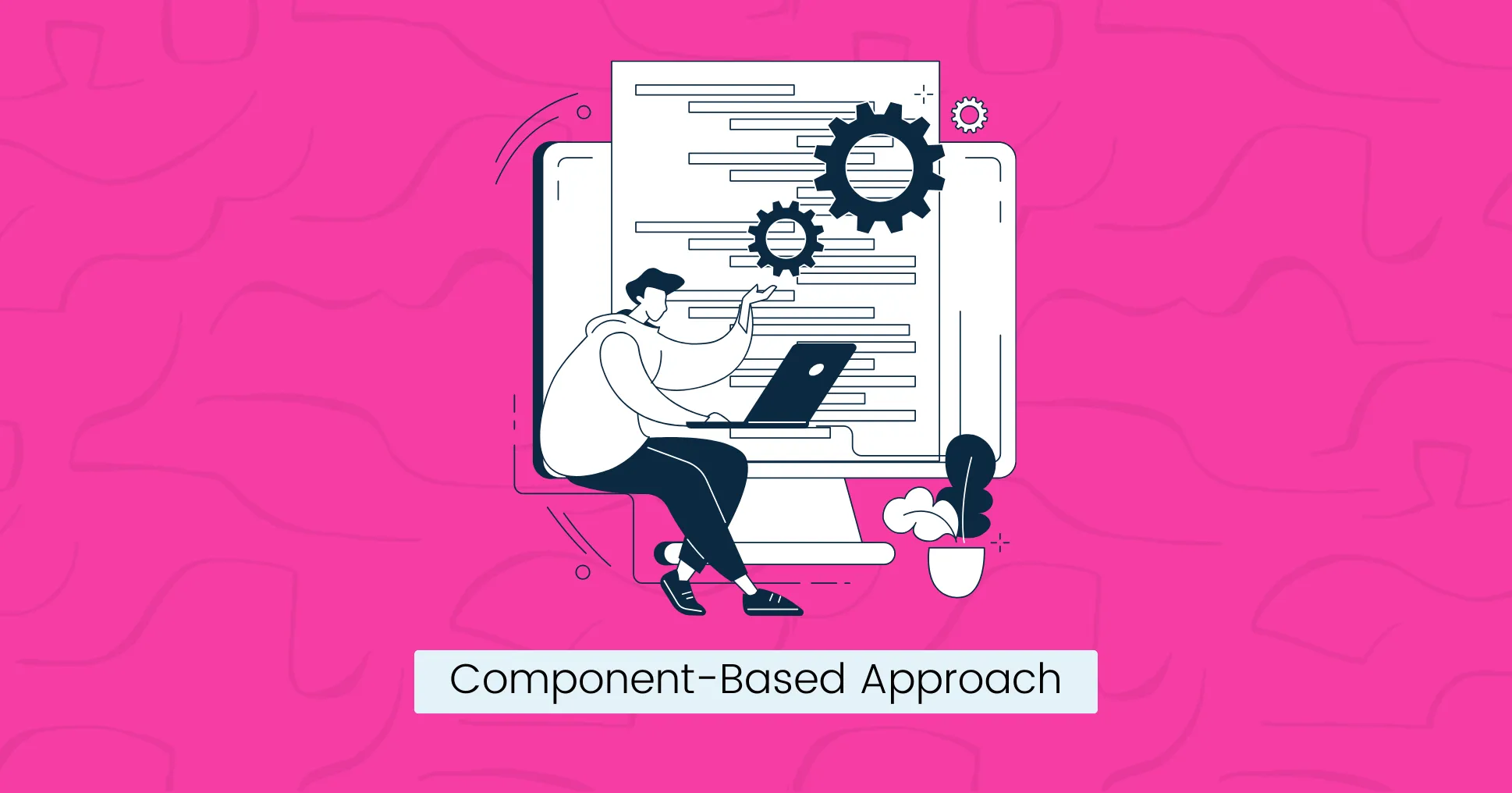
Imagine working on a single, large codebase. If you make changes to one feature, another part stops working.
Adopting a component-based approach can help you solve this problem.
This method involves breaking down software into smaller, reusable, and pre-defined components.
Each component represents a specific function and can be built and tested independently, making the overall system more modular and manageable.
Components can also be reused across different applications or projects.
This has many advantages, such as:
- Increases developer’s productivity.
- It speeds up development.
- Provides consistency and reduces errors.
- Creates scalable and maintainable software.
Test-Driven Development
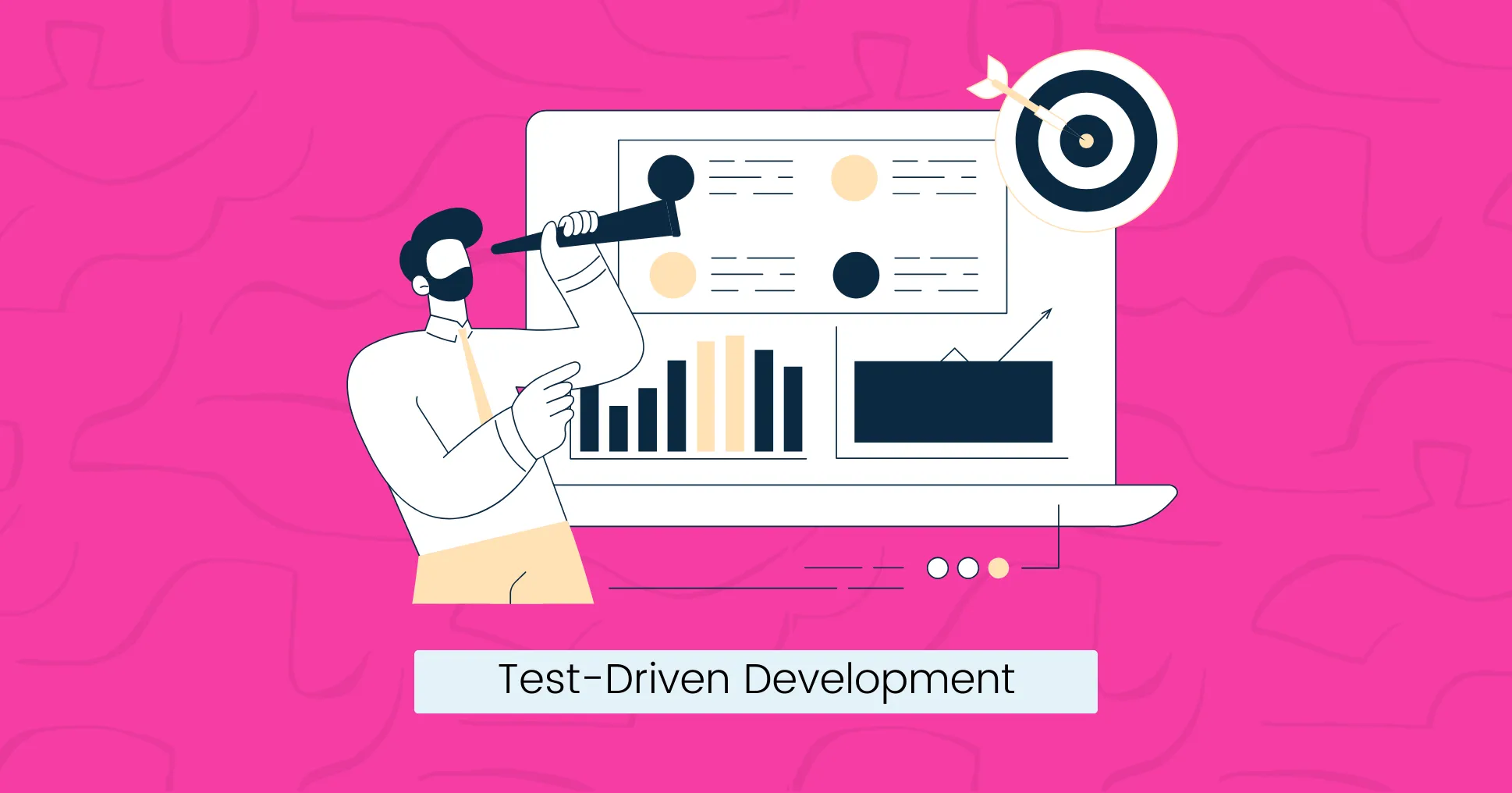
Testing is an essential part of the software development lifecycle.
You want your website to function properly after release to provide a great user experience.
When you develop software without a solid testing strategy, it can lead to:
- Frequent bugs and errors.
- Performance issues.
- Unpredictable behavior.
Fixing these issues later in the development process can be time-consuming and costly.
By writing tests first, you can verify that your application works as expected from the start.
Test-driven development has many benefits:
- Improve design and functionality based on software requirements specifications.
- Catch bugs early to minimize the cost and effort of fixing issues later.
- With a comprehensive test suite, you can confidently make changes without the fear of breaking existing functionality.
SOLID Design Principles
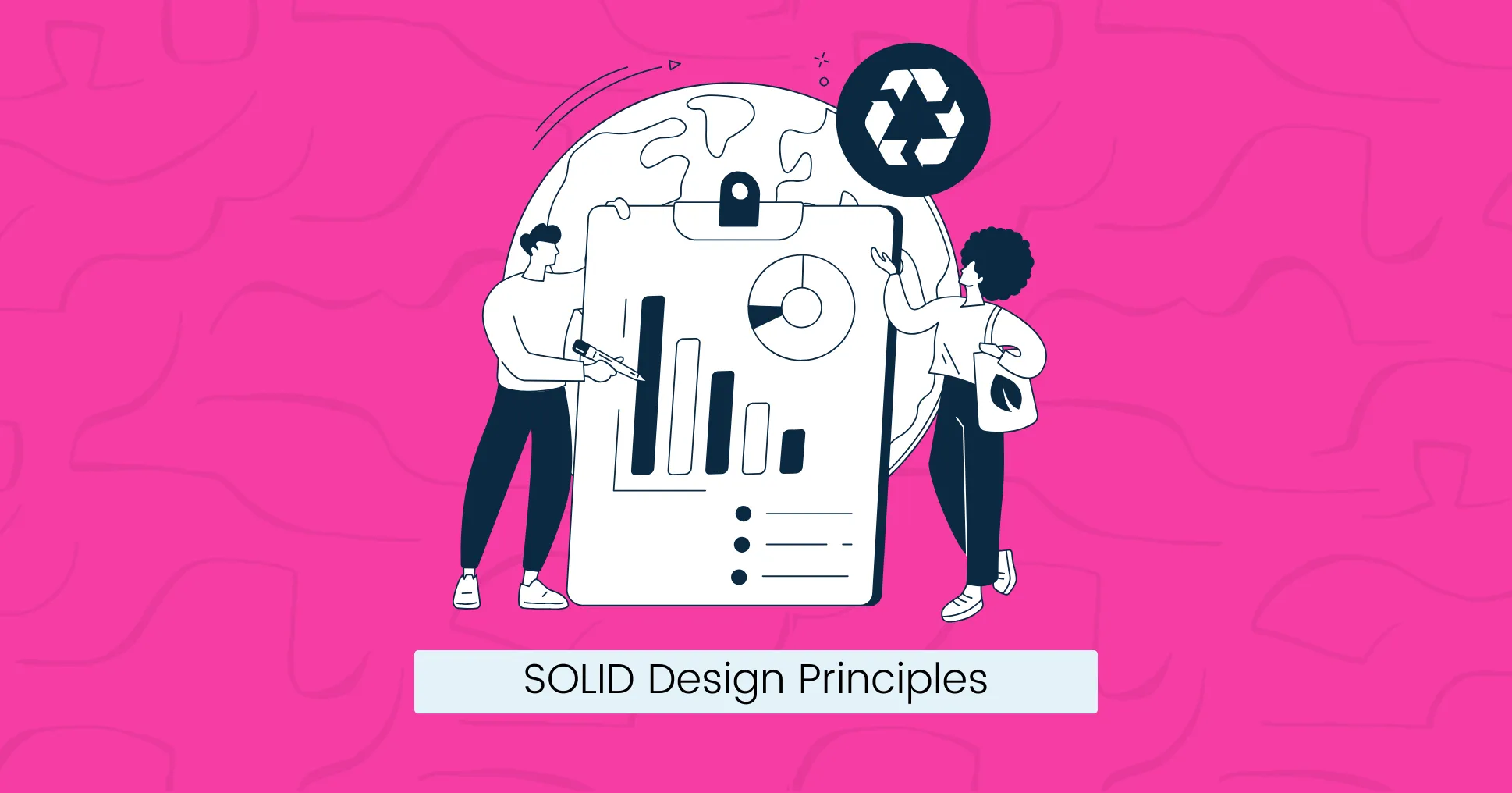
SOLID represents a set of five software design principles in object-oriented programming that help create more flexible and maintainable software solutions.
- Single Responsibility Principle (SRP): Each class should have a task or function. This makes it easier to avoid responsibilities becoming coupled.
- Open/Closed Principle (OCP): Software entities such as classes, modules, and functions should be open for extension but closed for modification. This allows developers to add new features without changing existing code.
- Liskov Substitution Principle (LSP): Subclass should be replaceable with its base class without affecting functionality.
- Interface Segregation Principle (ISP): Interfaces should be specific to client needs. Instead of creating a single, general-purpose interface, make multiple smaller, more targeted ones.
- Dependency Inversion Principle (DIP): Developers should depend on interfaces instead of implementations. This separates high-level and low-level modules, making the code easier to maintain.
snappify will help you to create
stunning presentations and videos.
Final Words
Software development principles help developers productively create more efficient systems.
By implementing these principles, you can develop flexible software capable of handling complex and changing requirements.
Follow best practices to improve your development process and deliver top-quality software faster.
If you like this article, you will also enjoy reading:
- Rubber duck debugging.
- How to become an AI engineer without a degree.
- How to become a 10x developer.
FAQs:
How can I implement software development principles into practice?
You can integrate software development principles into your development workflows, coding practices, and project management strategies for easy design and implementation.
What are the first principles of software testing?
The first principle of software testing is to validate software’s correctness, reliability, and usability through strategic and detailed evaluation.