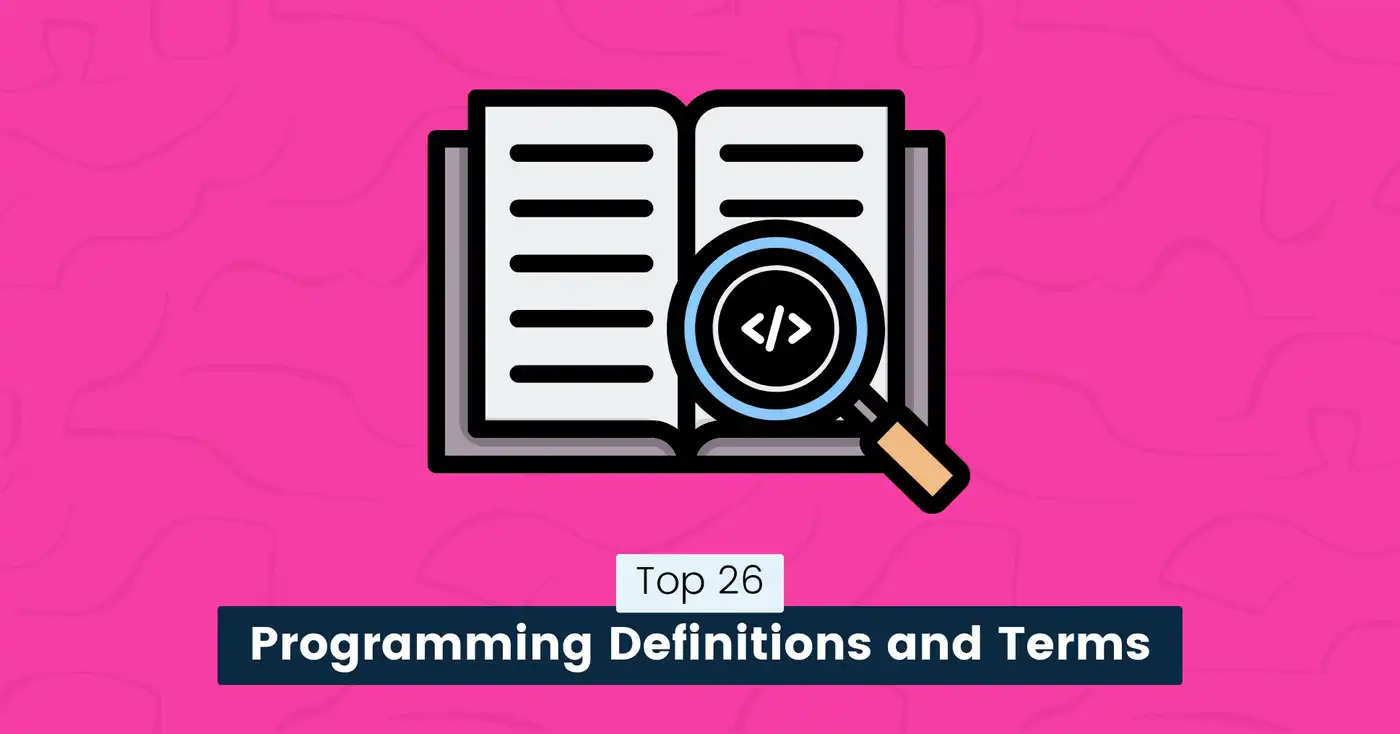
Top 26 Programming Definitions and Terms for Developers (2024)
The first step in acquiring any new skill is to become familiar with the terminology before practicing.
Understanding basic programming definitions is essential to grasp the domain and learn how developers create stunning applications from a piece of code.
In this article, you will learn the essential programming definitions and terms to help you build a solid foundation for your coding journey.
Programming Definitions and Terms
Computer programming or software development (coding) is how developers create and design executable computer programs to accomplish a specific task.
Programming includes activities such as:
- problem-solving
- algorithm design
- debugging
As a programmer, you need to:
- have a clear understanding of the problem
- break it down into smaller, manageable tasks
- then write code to implement the solution
Tip: A great way to learn any technology is by sharing what you learn. Sharing and explaining code snippets from your learning process will help you learn faster and better. Snappify is a tool that can help you create beautiful code snippets in minutes that your developer audience will love.
Here’s a list of some coding terminology you should know:
Program
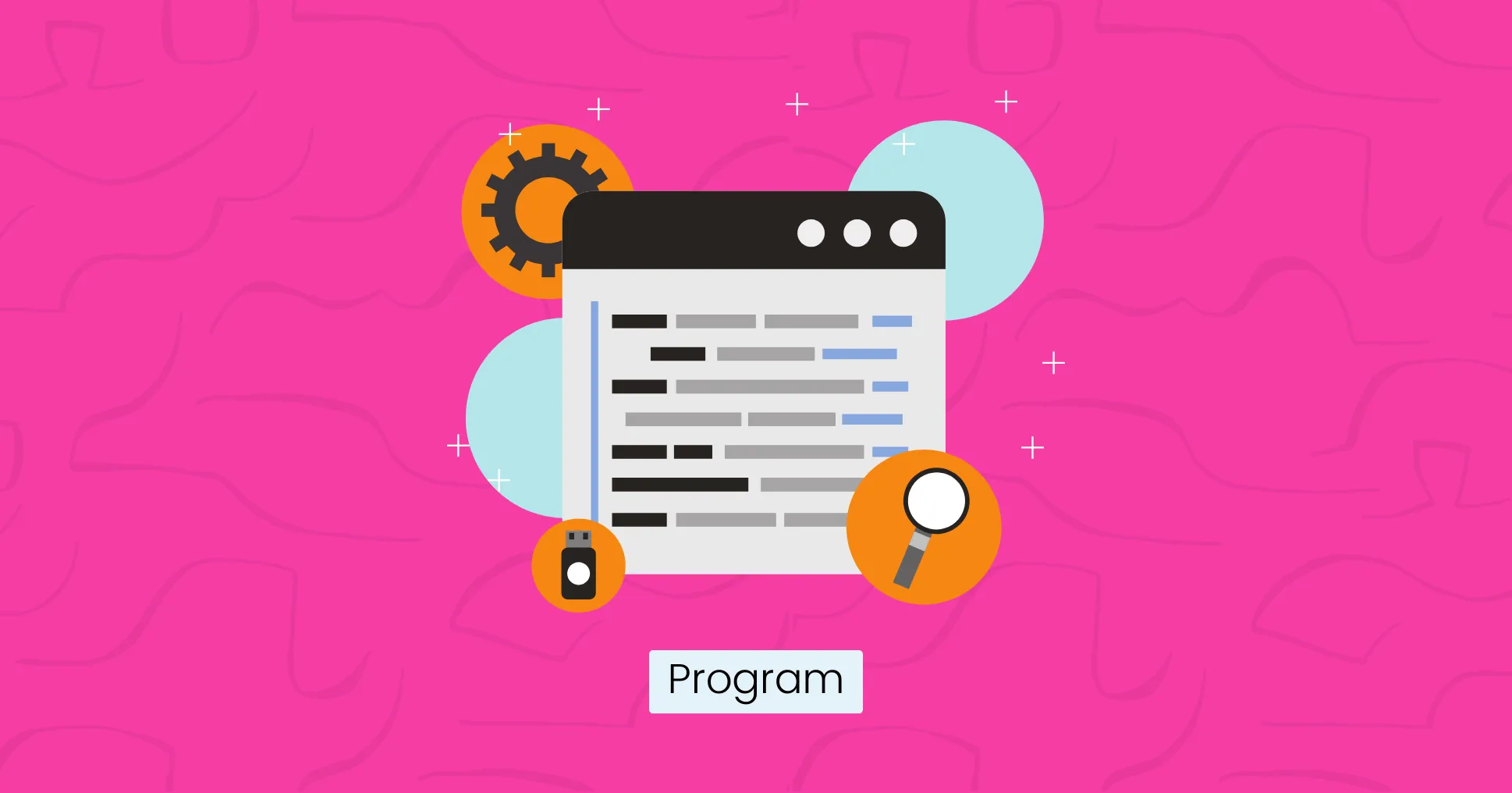
A computer program is a set of instructions designed to perform a specific task or function on a computer.
Some examples of computer programs are:
- Web browsers like Chrome or Firefox.
- Operating systems such as Windows or macOS.
- Development tools: Visual Studio Code.
- Games like Minecraft and Fortnite.
- Calculator Application
Algorithm
An algorithm is a step-by-step procedure or set of rules for solving a problem in programming.
For example, search engines like Google use algorithms to rank and retrieve information from extensive datasets.
Debugging
Debugging is the process of finding and fixing errors or bugs in a program.
The debugging process includes the following steps:
- Run the program and observe any errors.
- Review the code and locate the problem.
- Inspect the values of variables.
- Modify the code to address the issue.
API
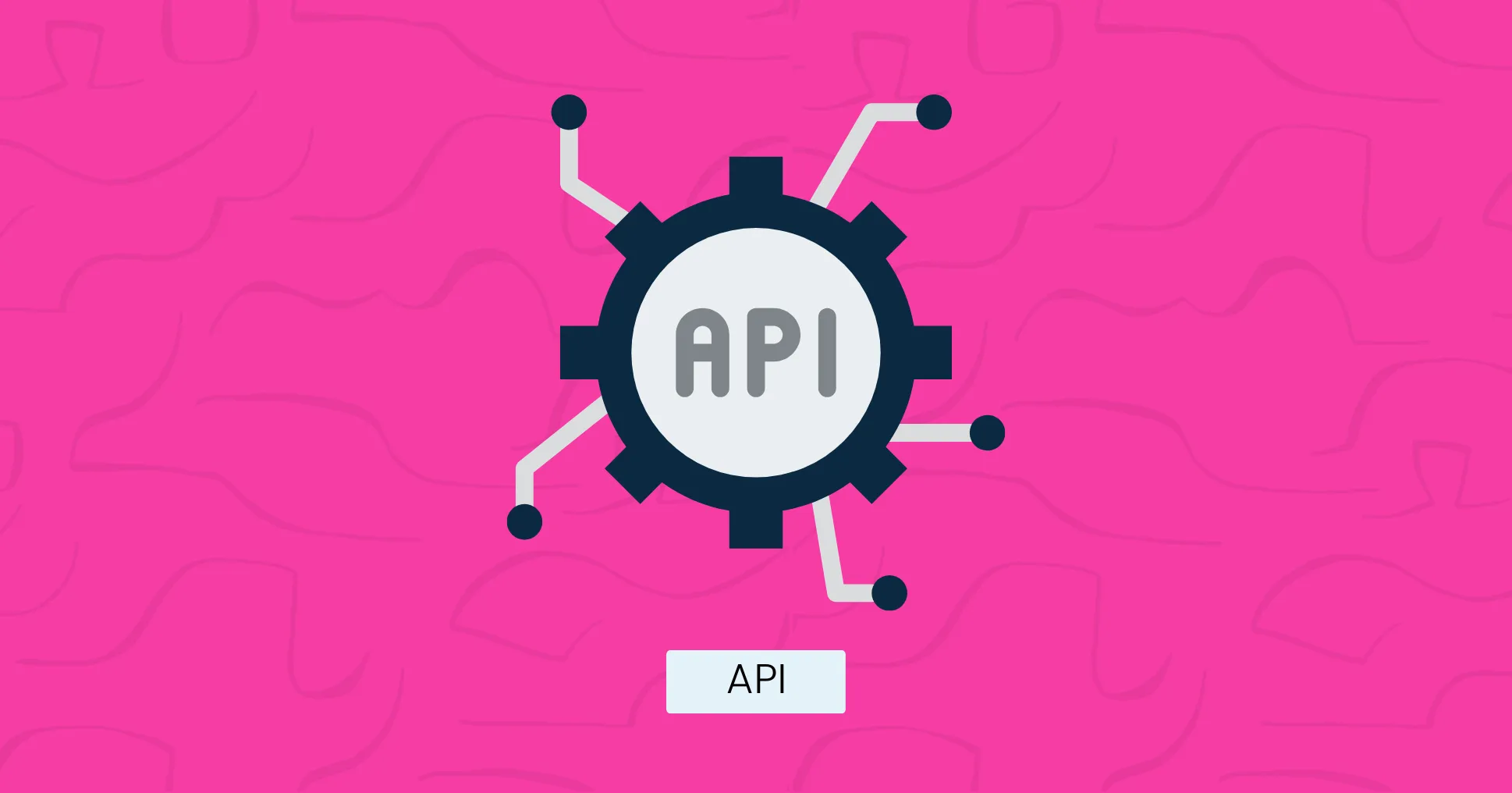
Application Programming Interface (API) is a set of rules, protocols, and tools for building software applications.
APIs define the interaction between different software components.
APIs can be used to integrate third-party services or data into your application, allowing you to use their functions without the need to build it from scratch.
Database
A database is a structured data collection organized for efficient retrieval and manipulation by a computer program.
It is like a central repository of archiving data for easier access.
Databases use a database management system (DBMS) for easier data maintenance.
Syntax
In programming languages, syntax defines the rules that control the formation of valid statements and expressions using a combination of symbols, keywords, and other elements.
It specifies the format and syntax of a programming language to ensure that the compiler or interpreter can understand it.
Keyword
A keyword is a word with a specific meaning used to define a program’s structure and logic.
It is a part of the syntax of a programming language.
A keyword has a reserved meaning in the language you can not redefine.
Some examples of keywords are if, else, while, for, float, class, and return.
Compilation
Compilation is a process by which developers can convert source code that is readable by humans into machine-readable code that a computer can run.
The software used for compiling is called a compiler.
Compilation is an important step in the execution of many programming languages. Popular programming languages like C, C++, and Java undergo compilation before execution.
Git
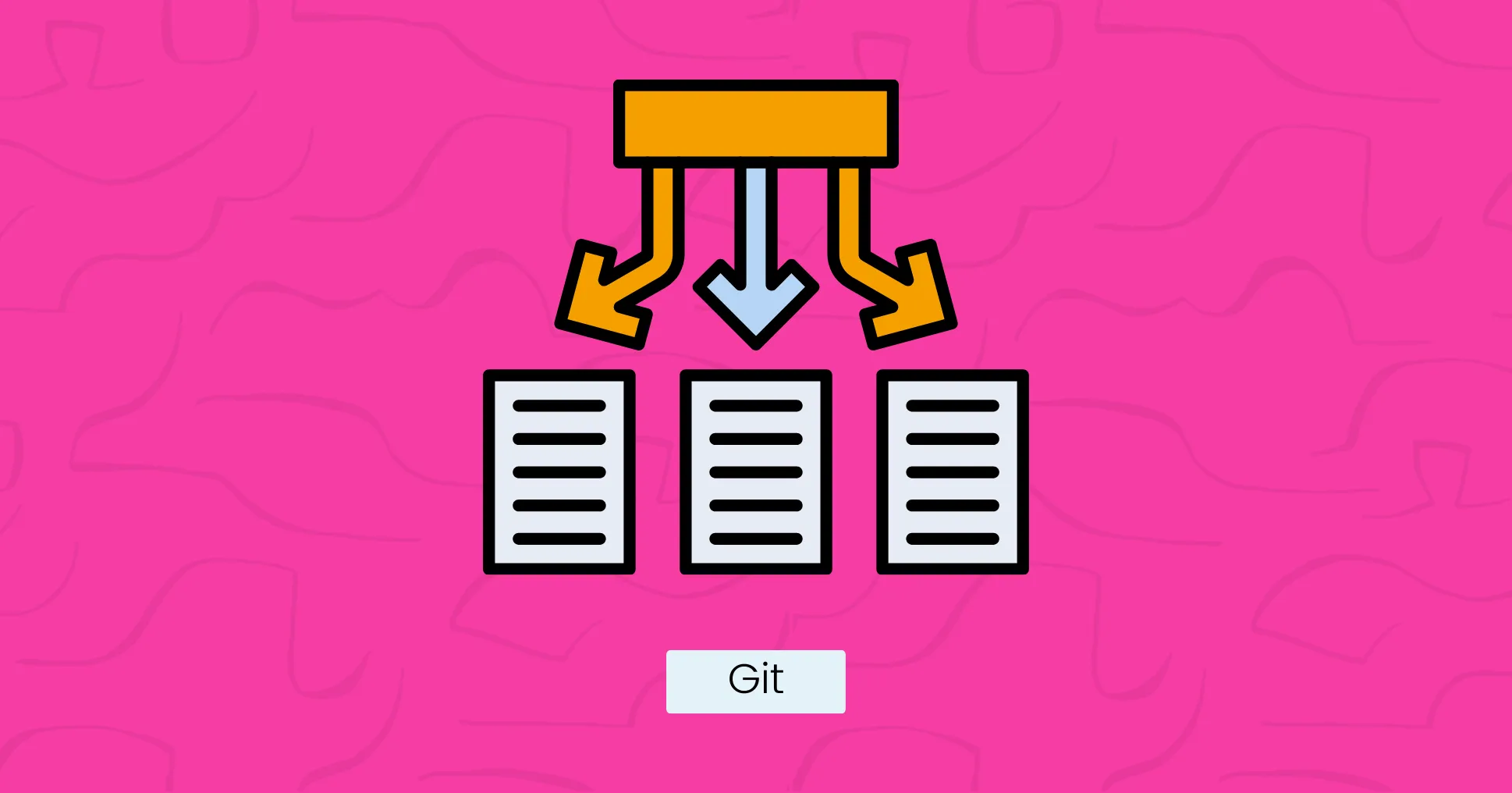
Git is a software used to track changes in source code during software development.
It is a distributed version control system that provides a full version history of the code.
Version control, also known as source control, is a system that manages changes to source code and tracks the history of those changes over time.
It allows multiple developers to work on the same codebase simultaneously while maintaining code quality.
Some popular version control solutions are:
- GitHub
- Bitbucket
Bug
A bug is an error, flaw, or unexpected behavior in a program that causes it to malfunction.
Bugs can occur due to mistakes during coding and cause inaccurate results.
Bug detection and fixing are essential parts of software development.
Common programming errors include syntax, logic, runtime, and semantic errors.
Runtime:
The period when an executable program runs on a system following its launch by a user or another program.
This phase includes:
- The program’s interaction with the system resources and user.
- Dynamic memory allocation and management.
- The execution of the program’s instructions by the CPU.
Build Time:
The time a program is constructed from code into an executable format by a compiler, linker, or similar build tools.
It is the phase where:
- Source code is translated into machine code or intermediate code.
- Dependencies are included and resolved.
- The final software package or binary is assembled.
Integrated Development Environment
An Integrated Development Environment (IDE) is a software application that programmers use for software development.
It includes a code editor, debugger, compiler, and other tools to facilitate development.
Popular IDEs for programming languages include:
- Visual Studio Code (a lightweight code editor)
- Visual Studio (for languages like C#, C++)
- Eclipse (for Java, C++)
- PyCharm (for Python)
Object-Oriented Programming
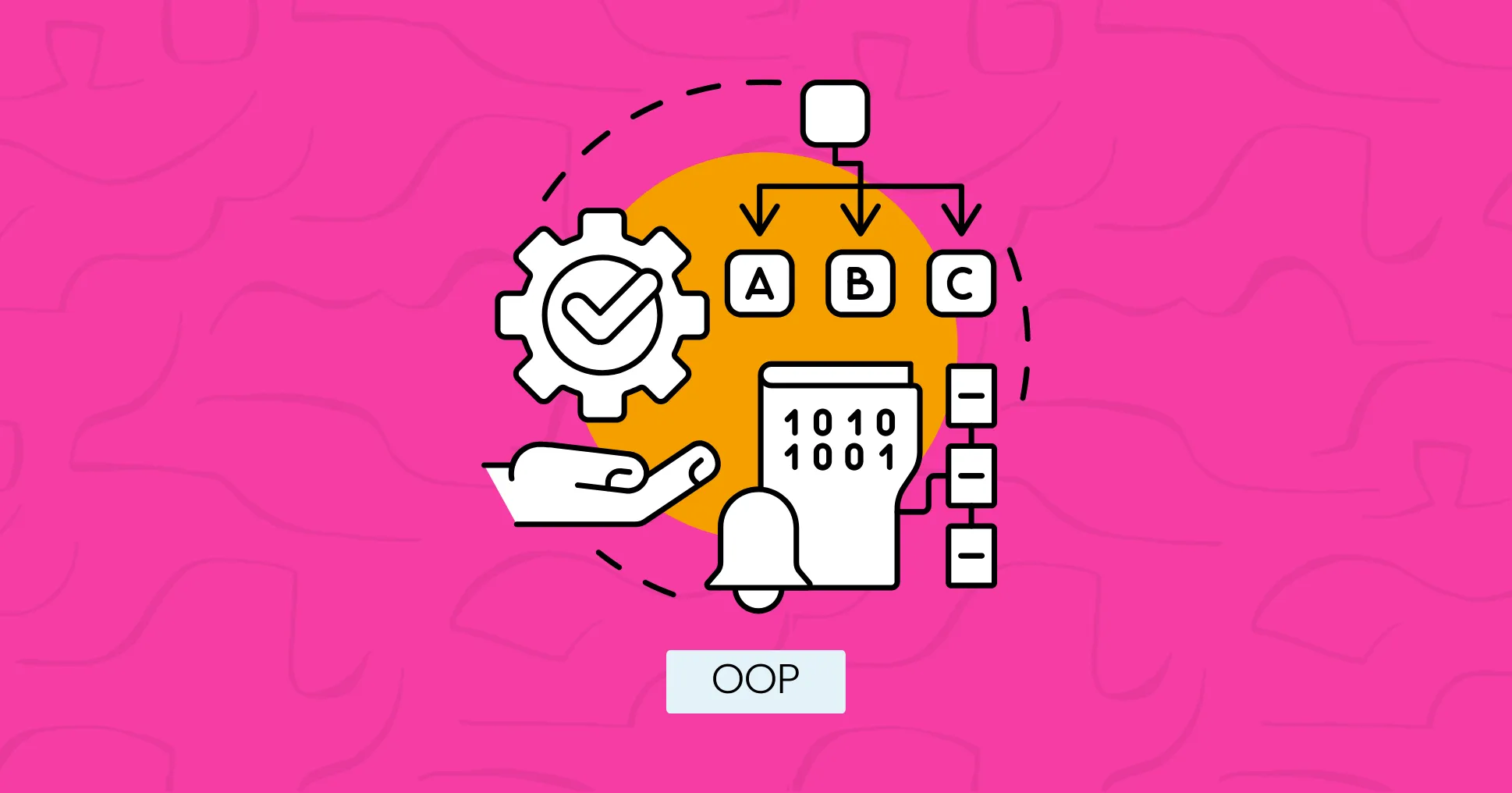
Object-Oriented Programming (OOP) is a programming model that uses objects or classes to design and organize code.
OOP is based on the concept of “objects,” which can encapsulate data (attributes) and behavior (methods).
With the help of OOP, you can structure code in a modular, reusable, and understandable way.
Class
In Object-oriented programming, a class is a template for creating objects. It defines the attributes and behaviors of the objects to separate them based on their characteristics.
A class can inherit properties and behaviors from another class, which promotes code reuse and forms a hierarchy between classes.
Programming Language
A programming language is a set of rules and syntax programmers use to write source code.
A compiler then translates The source code into machine language, enabling the computer to execute the program.
Some programming languages are:
- C++
- Java
- Python
- JavaScript
- Ruby
- PHP
Machine Language
Machine language is the binary code a computer’s CPU can execute directly. It consists of binary instructions, represented by 0 and 1, which correspond to specific operations performed by the hardware.
Conditionals
In programming, Conditionals are statements that allow code execution based on whether a specified condition is True or False.
Conditional statements help control the flow of a program, allowing it to make decisions based on the programmer’s needs.
HTTP/HTTPS
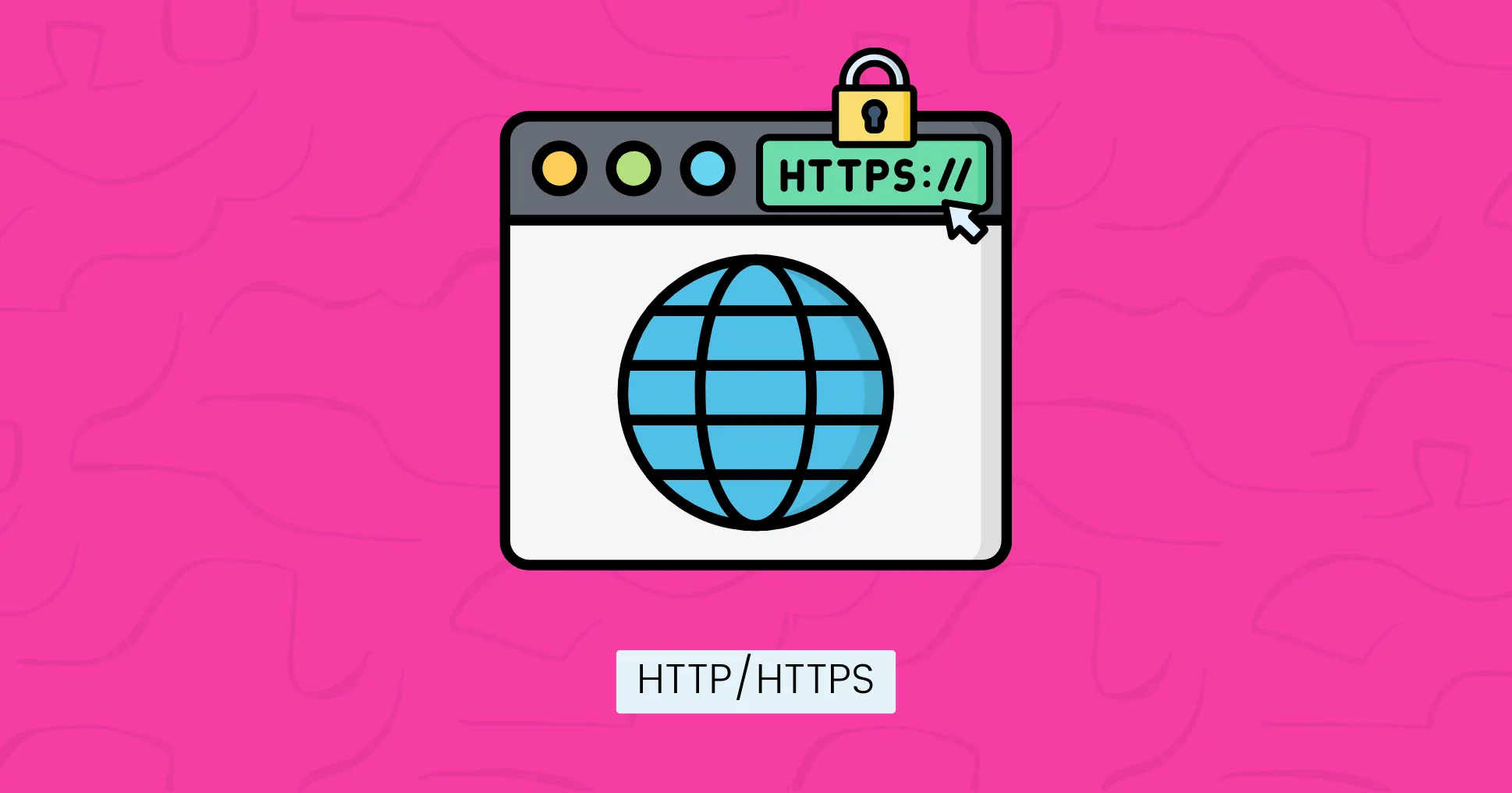
Hypertext Transfer Protocol or HTTP is an application-layer protocol that enables the transfer of HTML files over the internet.
It is the base of data communication on the World Wide Web.
HTTPS (HTTP Secure) is a secure version of HTTP that uses encryption (TLS/SSL protocols) to protect the data transmitted between client and server.
Frontend Development
Frontend development is the client-side development of a website or web application’s user interface and user experience.
Frontend developers work on designing the layout, visual elements, and features of a website that users interact with directly in their web browsers.
3 Core languages used in frontend development are:
- HTML (HyperText Markup Language): to create web page content like headings, paragraphs, links, and images.
- CSS (Cascading Style Sheets): for styling and layout like colors, spacing, fonts, etc.
- JavaScript: for interactive client-side behavior.
Backend Development
Backend development is the server-side development of a web application.
Backend developers focus on implementing the server logic, databases, APIs, and other components that enable the functionality of a website or web application.
Programming languages used in backend development are:
- Python
- Java
- PHP
- Ruby
UI Design
User Interface (UI) design refers to a website’s visual and interactive elements that users interact with directly.
It includes components such as:
- Layout
- Typography
- Colors
- Icons and images
- Buttons and links
UX Design
User Experience (UX) design is a user’s overall experience while interacting with a website.
It includes aspects of user interaction such as:
- Accessibility
- Performance
- Aesthetics
Framework
A web development framework is a reusable set of tools, libraries, and pre-written code that provides a foundation for building software applications.
It streamlines the development process and offers a structured way to create and organize code, reducing the need to write repetitive code.
Frameworks in different programming languages are:
- Django (Python)
- Ruby on Rails (Ruby)
- Laravel (PHP)
Some popular JavaScript frameworks are NextJS, React, and Angular.
Block Coding
Block coding is a specific part of a computer program.
It is a visual programming language where blocks are used instead of text.
It makes programming more accessible and introduces coding basics such as sequences, loops, and conditionals without requiring knowledge of syntax.
FAQs:
How can these coding terms and definitions be useful for a beginner programmer?
Understanding these programming terms and definitions is essential for beginners as it allows them to code accurately and efficiently. It helps them to understand the framework upon which the coding language operates, reducing the likelihood of stylistic and programming errors.
What is a Code Review?
A Code review is a systematic examination of computer source code intended to find and fix mistakes overlooked in the initial development phase, improving the overall quality of software and the developer’s skills.
Can you explain the term "CSS" in terms of coding terminology?
CSS stands for Cascading Style Sheets. It’s a stylesheet language used for describing the presentation of a document written in HTML.